Development/Tutorials/Qt4 Ruby Tutorial/Chapter 01
Tutorial Series | Qt4 Ruby Tutorial |
Previous | Ruby |
What's Next | Tutorial 2 - Calling it Quits |
Further Reading | n/a |
Hello World!
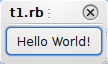
Files:
Overview
This first program is a simple "Hello world" example. It contains only the bare minimum you need to get a Qt application up and running. The picture above is a screenshot of this program.
Here's the complete source code for the application:
require 'Qt4'
app = Qt::Application.new(ARGV)
hello = Qt::PushButton.new('Hello World!')
hello.resize(100, 30)
hello.show()
app.exec()
Intro
The top level in a QtRuby application usually only needs to perform some kind of initialization and then pass control to the Qt library, which then tells the program about the user's actions via events.
There has to be exactly one Qt::Application object in every GUI application that uses Qt. Qt::Application manages various application-wide resources, such as the default font and cursor.
Qt::PushButton is a GUI push button that the user can press and release. It manages its own look and feel, like every other Qt::Widget. A widget is a user interface object that can process user input and draw graphics. The programmer can change both the overall look and feel and many minor properties of it (such as color), as well as the widget's content. A Qt::PushButton can show either text or a Qt::Icon.
Line by Line Walkthrough
require 'Qt4'
This line loads the QtRuby extension.
app = Qt::Application.new(ARGV)
app is this program's Qt::Application instance. It is created here. We pass ARGV to the Qt::Application constructor so that it can process certain standard command-line arguments (such as -display under X11). All command-line arguments recognized by Qt are removed from ARGV.
Note: It is essential that the Qt::Application object be created before any window-system parts of Qt are used.
hello = Qt::PushButton.new('Hello World!')
Here, after the Qt::Application, comes the first window-system code: A push button is created.
The button is set up to display the text "Hello world!". Because we don't specify a parent window (as second argument to the Qt::PushButton constructor), the button will be a window of its own, with its own window frame and title bar.
hello.resize(100, 30)
The button is set up to be 100 pixels wide and 30 pixels high (excluding the window frame, which is provided by the windowing system). We could call Qt::Widget::move() to assign a specific screen position to the widget, but instead we let the windowing system choose a position.
hello.show()
A widget is never visible when you create it. You must call Qt::Widget::show() to make it visible.
app.exec()
This is where our program passes control to Qt. Qt::CoreApplication::exec() will return when the application exits. (Qt::CoreApplication is Qt::Application's base class. It implements Qt::Application's core, non-GUI functionality and can be used when developing non-GUI applications.)
In Qt::CoreApplication::exec(), Qt receives and processes user and system events and passes these on to the appropriate widgets.
You should now try to run this program.
Running the Application
When you run the application, you will see a small window filled with a single button, and on it you can read the famous words: "Hello world!"
Exercises
Try to resize the window. Click the button. If you're running X11, try running the program with the -geometry option (for example, -geometry 100x200+10+20).