Development/Tutorials/First program (ko)
Tutorial Series | Beginner Tutorial |
Previous | C++, Qt, KDE4 development environment |
What's Next | 튜트리얼 2 - KXmlGuiWindow |
Further Reading | CMake |
개요
당신의 첫번째 프로그램은 "Hello World"로 친숙한 세계와의 인사가 될 것이다. 그밖에 무엇이 있겠는가? 그것을 위해, 우리는 KMessageBox와 커스텀마이징된 버튼들을 사용할 것이다.
- Your first program shall greet the world with a friendly "Hello World", what else? For that, we will use a KMessageBox and customise one of the buttons.
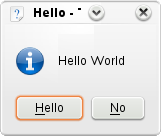
- tip|To get more information about any class you come across, Konqueror offers a quick shortcut. So to look for information about KMessageBox, just type "kde:kmessagebox" into Konqueror and you'll be taken to the documentation.
이 태스크를 위해 KDevelop를 설정하려면 이 튜트리얼을 읽어라.당신은 이미 존재하는 KDE4 어플리케이션을 KDevelop로 먼저 열기를 테스팅함으로써, 설정이 잘 동작하는지를 검사하기를 원할 것이다.
당신은 여전히 직접 CMake 파일들을 수정해야 한다.
- tip|
You might want to use KDevelop for your projects, which does many nice things like code completion, easy access to API documentation or debugging support.
Read this tutorial to set up KDevelop correctly for this task. You probably want to check if the setup is working by testing opening an existing KDE 4 application with KDevelop first.
You still need to edit the CMake files by hand though.
코드
당신이 원하는 모든 코드는 main.cpp 하나의 파일에 들어있다. 아래와 같은 코드 파일을 생성하자.
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
#include <KMessageBox>
int main (int argc, char *argv[])
{
KAboutData aboutData("tutorial1", // 내부적으로 사용하는 프로그램 이름
0, // 메세지 카탈로그 이름.
// 만약 null이면 프로그램 이름을 사용한다.
ki18n("Tutorial 1"), // 보여지는 프로그램 이름 문자열.
"1.0", // 프로그램 버전 문자열.
ki18n("KMessageBox popup"), // 프로그램이 하는 일에 대한 간단한 설명.
KAboutData::License_GPL, // 라이센스 식별자
ki18n("(c) 2007"), // Copyright 상태
ki18n("Some text..."), // 어느 정보나 포함하는 텍스트 자유폼.
"http://tutorial.com", // 프로그램 홈페이지 문자열.
"[email protected]"); // 버그 리포트 이메일 주소 문자열.
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
KGuiItem guiItem( QString( "Hello" ), QString(),
QString( "this is a tooltip" ),
QString( "this is a whatsthis" ) );
KMessageBox::questionYesNo( 0, "Hello World", "Hello", guiItem );
}
처음 프로그램에서 만나는 KDE 코드는 KAboutData이다. 이것은 짧은 설명, 저자, 라이센스 정보와 같은 프로그램에 관한 정보를 저장한다. 꽤 많은 KDE 어플리케이션이 이 클래스를 사용해야 한다.
- The first KDE specific code we come across in this program is KAboutData. This is the class used to store information about the program such as a short description, authors or license information. Pretty much every KDE application should use this class.
그러면 KCmdLineArgs을 살펴보자. 이것은 누군가가 특정 커맨드라인 스위치를 사용하기 위한 클래스이다. 예를 들어 특정 파일을 프로그램으로 열기. 그러나 이 튜트리얼에서는 간단하게 이것을 우리가 생성한 KAboutData 오브젝트로 초기화한다. 그래서 우리는 --version과 ----author 스위치를 사용할 수 있다.
이제 우리는 KApplication 오브젝트를 생성한다. i18n.
과 같은 것들이 필요할 때로부터, 이것은 정확하게 각각의 프로그램에서 한 번 수행되는 것이 필요하다.
- Then we create a KApplication object. This needs to be done exactly once in each program since it is needed for things such as i18n.
이제 우리는 필수적인 모든 KDE 설정을 수행하였다. 우리는 우리의 어플리케이션이 수행하는 흥미로운 쪽으로 이동할 수 있다. 이제 팝업 박스를 생성할 것이다. 그러나, 우리는 버튼을 커스텀마이징 해야한다.버튼을 커스텀마이징하기 위해서, 우리는 KGuiItem 오브젝트를 사용할 필요가 있다. KGuiItem 생성자의 첫번째 인자는 아이템에서 나타날 텍스트이다.(우리의 경우 버튼). 그러면 우리는 버튼을 위한 아이콘을 설정하는 옵션이 있지만, 우리는 그것을 원하지 않기 때문에, QString()으로 주었다. 마지막으로 툴팁(item에 마우스를 위에 놔두었을 때 나타나는)과 "What's This?" 텍스트(오른쪽 버튼 클릭 또는 Shift-F1) 텍스트를 설정하였다.
- Now we've done all the necessary KDE setup, we can move on to doing interesting things with our application. We're going to create a popup box but we're going to customise one of the buttons. To do this customisation, we need to use a KGuiItem object. The first argument in the KGuiItem constructor is the text that will appear on the item (in our case, a button). Then we have an option of setting an icon for the button but we don't want one so we just give it QString(). Finally we set the tooltip (what appears when you hover over an item) and finally the "What's This?" (accessed through right-clicking or Shift-F1) text.
이제 아이템을 가졌으며, 팝업을 생성할 수 있다. 이제 우리는 기본적으로 하나의 "Yes"와 하나의 "No" 버튼을 가진 메세지 박스를 생성하는 KMessageBox::questionYesNo()함수를 호출한다. 두번째 인자는 팝업 박스의 중간에 나타날 텍스트이다. 세번째는 윈도우가 가지는 캡션이며, 마지막으로 우리는 KGuiItem을 (일반적인) "Yes" 버튼을 우리가 생성한 KGuiItem guiItem으로 설정한다.
우리는 코드와 관계된 모든 것을 살펴보았다. 이제 빌드하고 실행해야한다.
- Now we have our item, we can create our popup. We call the KMessageBox::questionYesNo() function which, by default, creates a message box with a "Yes" and a "No" button. The second argument is the text that will appear in the middle of the popup box. The third is the caption the window will have and finally we set the KGuiItem for (what would normally be) the "Yes" button to the KGuiItem guiItem we created.
We're all done as far as the code is concerned. Now to build it and try it out.
빌드
만약 Getting Started/Build/KDE4에서 설명한 것처럼 환경을 설정하였다면, 이 코드를 다음 과 같이 컴파일 할 수 있다.
- If you set up your environment as described in Getting Started/Build/KDE4, you can compile this code with
g++ main.cpp -o tutorial1 \ -I$QTDIR/include/Qt \ -I$QTDIR/include/QtCore \ -I$QTDIR/include \ -I$KDEDIR/include/KDE \ -I$KDEDIR/include \ -L$KDEDIR/lib \ -L$QTDIR/lib -lQtCore -lQtGui -lkdeui -lkdecore
그리고 이것을 다음과 같이 실행한다.
- and then run it with
dbus-launch ./tutorial1
CMake 사용하기
만약 위의 작업이 동작했다면, 우리는 KDE의 남은 부분과 같이 CMake를 사용하기를 원할 것이다. 이것은 KDE, Qt 그밖의 것들을 위한 헤더와 라이브러리를 자동적으로 지정할 것이다. 그리고, 쉽게 다른 컴퓨터에서 당신의 어플리케이션을 빌드할 수 있다.
- If that worked, you may want to use CMake, just like the rest of KDE. This will automatically locate the libraries and headers for KDE, Qt etc. and will allow you to easily build your applications on other computers.
CMakeLists.txt
main.cpp와 같은 디렉토리에 CMakeList.txt라는 이름의 파일을 생성한다.:
- Create a file named CMakeLists.txt in the same directory as main.cpp with this content:
project (tutorial1)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(tutorial1_SRCS main.cpp)
kde4_add_executable(tutorial1 ${tutorial1_SRCS})
target_link_libraries(tutorial1 ${KDE4_KDEUI_LIBS})
find_package() 함수는 당신이 요청한 (이 경우에는 KDE4) 패키지의 장소를 알아내고, 패키지의 헤더와 라이브러리의 장소를 설명하는 몇몇 변수를 설정한다. 이경우 우리는 KDE4 헤더 파일의 경로가 포함된 KDE4_INCLUDES 변수를 사용한다.
- The find_package() function locates the package that you ask it for (in this case KDE4) and sets some variables describing the location of the package's headers and libraries. In this case we will use the KDE4_INCLUDES variable which contains the path to the KDE4 header files.
이러한 파일들을 찾도록 컴파일러를 허가하기 위해, 우리는 이 변수를 헤더 검색 경로에 KDE4 헤더를 추가하는 include_directories()함수로 보낼수 있다.
- In order to allow the compiler to find these files, we pass that variable to the include_directories() function which adds the KDE4 headers to the header search path.
다음 우리는 set() 함수를 사용하여 tutorial1_SRCS라고 불리는 변수를 생성한다. 이경우, 우리는 간단히 오직 소스파일의 이름으로 설정하였다.
- Next we create a variable called tutorial1_SRCS using the set() function. In this case we simply set it to the name of our only source file.
이제 우리의 tutorial1_SRCS 변수에 리스트된 소스 파일에서부터 tutorial1이라 불리는 실행파일을 생성하기 위해 kde4_add_executable()를 사용한다. 마지막으로 우리의 실행파일을 target_link_libraries()를 사용하여 KDE4 kdeui 라이브러리와 find_package()함수로 설정된 KDE4_KDEUI_LIBS 변수와 함께 연결한다.
- Then we use kde4_add_executable() to create an executable called tutorial1 from the source files listed in our tutorial1_SRCS variable. Finally we link our executable to the KDE4 kdeui library using target_link_libraries() and the KDE4_KDEUI_LIBS variable which was set by the find_package() function.
Make And Run
Again, 만약 우리가 Getting Started/Build (ko)/KDE4 에서 설명된 환경을 설정하였다면, 다음과 같이 컴파일 할 수 있다.:
- Again, if you set up your environment as described in Getting Started/Build/KDE4, you can compile this code with:
cmakekde
또는 만약 설정하지 않았다면:
- Or if you did not:
mkdir build && cd build cmake .. make
그리고 다음과 같이 실행한다.:
- And launch it with:
./tutorial1
나아가기
이제 당신은 KXmlGuiWindow 사용하기로 나아갈 수 있다.
- Now you can move on to using KXmlGuiWindow.