Development/Tutorials/Using KActions (ko): Difference between revisions
Neverendingo (talk | contribs) m (Text replace - "</code>" to "</syntaxhighlight>") |
Neverendingo (talk | contribs) m (Text replace - "<code xml n>" to "<syntaxhighlight lang="xml" line>") |
||
Line 202: | Line 202: | ||
===tutorial3ui.rc=== | ===tutorial3ui.rc=== | ||
< | <syntaxhighlight lang="xml" line> | ||
<?xml version="1.0" encoding="UTF-8"?> | <?xml version="1.0" encoding="UTF-8"?> | ||
<gui name="tutorial3" | <gui name="tutorial3" |
Revision as of 21:04, 29 June 2011
Development/Tutorials/Using_KActions
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Beginner Tutorial |
Previous | 튜트리얼 2 - KXmlGuiWindow, 기본 xml 지식 |
What's Next | 튜트리얼 4 - Saving and loading |
Further Reading | None |
개요
이 튜트리얼은 액션의 개념에 대하여 소개한다. 액션은 우리의 프로그램과 상호작용하는 방식을 유저에게 지원하는 통합된 방법이다.
- This tutorial introduces the concept of actions. Actions are a unified way of supplying the user with ways to interact with your program.
예를 들어 튜트리얼 2 에서 유저가 툴바 안의 버튼이나 파일메뉴의 옵션을 클릭하거나, 키보드 단축키를 통하여 텍스트 박스의 내용을 지우기를 원한다면, 하나의 KAction을 통하여 모든 것이 가능하도록 할 수 있다.
- For example, if we wanted to let the user of Tutorial 2 clear the text box by clicking a button in the toolbar, from an option in the File menu or through a keyboard shortcut, it could all be done with one KAction.
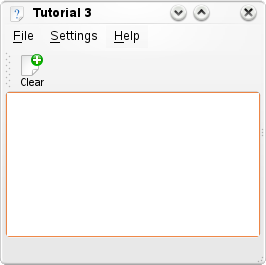
KAction
KAction은 특정 액션과 관계된 아이콘과 단축키에 대한 모든 정보를 포함하는 오브젝트이다. 액션은 액션의 작업을 수행하는 슬롯과 연결되어져 있다.
- A KAction is an object which contains all the information about the icon and shortcuts that is associated with a certain action. The action is then connected to a slot which carries out the work of your action.
The Code
main.cpp
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
#include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial3", "tutorial3",
ki18n("Tutorial 3"), "1.0",
ki18n("A simple text area using KAction etc."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
이번에는 main.cpp에서 아주 조금만 바뀌었다. 단지 KAboutData 생성자가 tutrial3으로 보여지도록 업데이트 되었을 뿐이다.
- This time, very little has changed in main.cpp, only the KAboutData constructor has been updated to show that we are now on tutorial 3.
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <KXmlGuiWindow>
#include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
void setupActions();
};
#endif
void setupActions()함수는 단지 KActions을 설정하는 모든 작업을 수행하도록 추가되어진다.
- Only a function void setupActions() has been added which will do all the work setting up the KActions.
mainwindow.cpp
#include "mainwindow.h"
#include <KApplication>
#include <KAction>
#include <KLocale>
#include <KActionCollection>
#include <KStandardAction>
MainWindow::MainWindow(QWidget *parent)
: KXmlGuiWindow(parent)
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KAction* clearAction = new KAction(this);
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("document-new"));
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
actionCollection()->addAction("clear", clearAction);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()),
actionCollection());
setupGUI();
}
설명
이것은 Tutorial 2부터 KXmlGuiWindow 코드로 작성한다. 대부분의 바뀐 부분은 mainwindow.cpp이다. 중요한 구조적 변화 중 하나는 MainWindow를 위해 setupGUI() 대신 setupAction()을 수행한 것이다. setupActions()는 마지막으로 스스로 setupGUI()는 호출하기 전에, KAction 코드를 생성하는 곳이다.
- This builds upon the KXmlGuiWindow code from Tutorial 2. Most of the changes are to mainwindow.cpp, an important structural change being that the constructor for MainWindow now calls setupActions() instead of setupGUI(). setupActions() is where the new KAction code goes before finally calling setupGUI() itself.
KAction 오브젝트 생성하기
The KAction은 몇 단계에 걸쳐서 생성된다. 처음으로 하는 일은 KAction 라이브러리를 추가하고 KAction을 생성하는 것이다.:
- The KAction is built up in a number of steps. The first is including the KAction library and then creating the KAction:
#include <KAction>
...
KAction* clearAction = new KAction(this);
이것은 clearAction이라고 불리는 하나의 새로운 KAction을 생성한다.
- This creates a new KAction called clearAction.
KAction 속성 설정하기
텍스트
이제 우리는 KAction 오브젝트를 가지며, KAction 속성을 설정하는 것을 시작할 수 있다. 다음 코드는 메뉴와 툴바의 KAction의 아이콘에서 보여질 텍스트를 설정한다.
- Now we have our KAction object, we can start setting its properties. The following code sets the text that will be displayed in the menu and under the KAction's icon in the toolbar.
clearAction->setText(i18n("Clear"));
이 텍스트가 i18n() 함수를 거쳤다는 것에 대하여 상기하라.; 이것은 UI에서 번역가능하도록 만들기 위해 필수적인 과정이다. (좀더 자세한 정보는 i18n 튜트리얼에서 찾을 수 있다.)
- Note that the text is passed through the i18n() function; this is necessary for the UI to be translatable (more information on this can be found in the i18n tutorial).
아이콘
만약 액션이 툴바에서 보여지게 하려면, 액션을 묘사하는 아이콘을 가지도록 하는 것이 좋다. 다음 코드는 KDE 표준 "document-new" 아이콘을 setIcon() 함수를 사용하여 설정한다. :
- If the action is going to be displayed in a toolbar, it's nice to have an icon depicting the action. The following code sets the icon to the standard KDE document-new icon through the use of the setIcon() function:
clearAction->setIcon(KIcon("document-new"));
키보드 단축키
액션을 수행하는 키보드 단축키를 설정하는 것은 간단하다.:
- Setting a keyboard shortcut to perform our action is equally simple:
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
이것은 Ctrl+W와 KAction과 연관시킨다.
- This associates Ctrl+W with the KAction.
Collection 추가하기
XMLGUI 프레임워크(나중에 좀더 깊게 설명되어진다.)에 의해 접근되어지는 액션이 차례대로 어플리케이션의 action collection에 추가되어져야 한다. action collection은 다음과 같이 actionCollection()를 통해 접근된다.:
- In order for the action to be accessed by the XMLGUI framework (explained in depth later) it must be added to the application's action collection. The action collection is accessed via the actionCollection() function like this:
actionCollection()->addAction("clear", clearAction);
여기 clearAction KAction은 collection에 추가되어지며, clear라는 이름으로 붙여진다. 이 이름(clear)은 액션을 참조하기 위해 XMLGUI 프레임워크이 사용한다.
- Here, the clearAction KAction is added to the collection and given a name of clear. This name (clear) is used by the XMLGUI framework to refer to the action.
액션 연결하기
이제 완벽히 액션을 설정하려면, 좀 더 유용하게 연결되어질 필요가 있다. 이 경우(텍스트 영역을 지우기를 원하므로), 우리는 KTextEdit이 가지고 있는 (놀랍게도 KTextEdit를 지우는)clear() 액션과 우리의 액션을 연결한다.
- Now that the action is fully set up, it needs to be connected to something useful. In this case (because we want to clear the text area), we connect our action to the clear() action belonging to a KTextEdit (which, unsurprisingly, clears the KTextEdit)
connect( clearAction, SIGNAL( triggered(bool) ),
textArea, SLOT( clear() ) );
이것은 Qt에서 QAction이 수행되는 것과 같다.
- This is the same as it would be done in Qt with a QAction.
KStandardAction
모든 KDE 어플리케이션은 대부분 'quit', 'save', 'load'와 같은 액션을 위해, KStandardAction을 통해 접근되는 미리 만들어진 편리한 KAction이 존재한다.
- For actions which would likely appear in almost every KDE application such as 'quit', 'save', and 'load' there are pre-created convenience KActions, accessed through KStandardAction.
이것은 사용하는 것은 간단하다. 라이브러리에 한번 include 되어지며(#include <KStandardAction>), 간단하게 당신이 수행하기를 원하는 함수와 함께 KActionCollection이 추가되도록 이것을 지원하라. 예를 들어:
- They are very simple to use. Once the library has been included (#include <KStandardAction>), simply supply it with what you want the function to do and which KActionCollection to add it to. For example:
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
이것은 적절한 아이콘, 텍스트, 단축키을 가진 KAction을 만들고, 파일 메뉴에도 추가한다.
- This creates a KAction with the correct icon, text and shortcut and even adds it to the File menu.
메뉴와 툴바에 액션 추가하기
잠깐동안 새로운 "Clear" 액션이 만들어진다. 그러나 이것은 어떠한 메뉴나 툴바에도 연관되어 있지 않다. 이것을 수행하는 것은 유동적인 XMLGUI로 불리는 KDE 기술이다.
- At the moment, the new "Clear" action has been created but it hasn't been associated with any menus or toolbars. This is done with a KDE technology called XMLGUI, which does nice things like movable toolbars for you.
- note|In a later version of KDE4, XMLGUI, may be replaced with a new framework called liveui. For now, XMLGUI, is the only and correct way to set up the UI.
XMLGUI
KXmlGuiWindow에서 setupGUI() 함수는 XMLGUI 시스템에 의존적이며, XMLGUI는 인터페이스 XML 파일을 파싱함으로써 GUI를 생성한다.
- The setupGUI() function in KXmlGuiWindow depends on the XMLGUI system to construct the GUI, which XMLGUI does by parsing an XML file description of the interface.
이 XML 파일에 이름을 붙이는 규정은 appnameui.rc이다. appname에는 KAboutData에서 설정한 이름(이 경우 tutorial3)이 들어간다. 우리의 경우, 이 파일은 tutorial3ui.rc으로 이름지어지며, 빌드 디렉토리에 위치하게 된다. 파일이 마지막으로 위치할 곳은 CMake에 의해 결정된다.
- The rule for naming this XML file is appnameui.rc, where appname is the name you set in KAboutData (in this case, tutorial3). So in our example, the file is called tutorial3ui.rc, and is located in the build directory. Where the file will ultimately be placed is handled by CMake.
appnameui.rc File
UI가 XML로 정의된 이후에 레이아웃은 엄격한 규칙에 따라야한다. 이 튜트리얼은 이 주제에 대하여 깊게 들어가지 않을 것이다. 그러나 좀더 정보가 필요하면, 세부화된 detailed XMLGUI page를 살펴보라.
- Since the description of the UI is defined with XML, the layout must follow strict rules. This tutorial will not go into great depth on this topic, but for more information, see the detailed XMLGUI page.
tutorial3ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<gui name="tutorial3"
version="1"
xmlns="http://www.kde.org/standards/kxmlgui/1.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.kde.org/standards/kxmlgui/1.0
http://www.kde.org/standards/kxmlgui/1.0/kxmlgui.xsd" >
<MenuBar>
<Menu name="file" >
<Action name="clear" />
</Menu>
</MenuBar>
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
</gui>
<Toolbar> 태그는 아이콘과 함께 일반적으로 윈도우의 상단에 위치하는 툴바를 묘사한다. 여기에서는 mainToolBar라는 독특한 이름이 붙여지며, 유저에게 보이는 이름은 <text> 태그를 사용하는 mainToolbar로 설정된다. clear 액션은 <Action> 태그를 사용하는 툴바에 추가되어지며, 이 태그의 name 파라미터는 mainwindow.cpp에서 addAction()으로 KActionCollection으로 넘겨지는 문자열이 된다.
- The <Toolbar> tag allows you to describe the toolbar, which is the bar across the top of the window normally with icons. Here it is given the unique name mainToolBar and its user visible name set to Main Toolbar using the <text> tag. The clear action is added to the toolbar using the <Action> tag, the name parameter in this tag being the string that was passed to the KActionCollection with addAction() in mainwindow.cpp.
툴바에서 가지고 있는 액션은 메뉴바에도 추가된다. 여기서 액션은 툴바에서 추가되었던 방식과 같은 방식으로MenuBar의 File 메뉴에 추가되어진다.
- Besides having the action in the toolbar, it can also be added to the menubar. Here the action is being added to the File menu of the MenuBar the same way it was added to the toolbar.
시스템 캐시를 업데이트하기 위한 마지막 설치 이후에, .rc 파일을 변경하였다면 <gui> 태그의 'version' 속성이 변경된다.
- Change the 'version' attribute of the <gui> tag if you changed .rc file since the last install to force a system cache update.
CMake
마지막으로 tutorial3ui.rc는 KDE가 찾을 수 있는 어딘가로 이동할 필요가 있다.(소스 디렉토리에서는 벗어나지 못한다!) 이것은 프로젝트가 어딘가로 옮겨질 필요가 있다는 것을 의미한다.
- Finally, the tutorial3ui.rc needs to go somewhere where KDE can find it (can't just leave it in the source directory!). This means the project needs to be installed somewhere.
CMakeLists.txt
project(tutorial3)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(tutorial3_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial3 ${tutorial3_SRCS})
target_link_libraries(tutorial3 ${KDE4_KDEUI_LIBS})
install(TARGETS tutorial3 DESTINATION ${BIN_INSTALL_DIR})
install(FILES tutorial3ui.rc
DESTINATION ${DATA_INSTALL_DIR}/tutorial3)
</syntaxhighlight>
이 파일은 대부분 튜트리얼 2에서 확인하였다. 확인하지 않았던 마지막의 두 줄은 파일을 어디에 설치할지를 설명한다. 먼저 튜트리얼 3 타겟은 BIN_INSTALL_DIR에 설치된다. 그리고 유저 인터페이스의 레이아웃을 묘사하는 tutorial3ui.rc 파일은 어플리케이션의 데이터 디렉토리에 설치된다.
- This file is almost identical to the one for tutorial2, but with two extra lines at the end that describe where the files are to be installed. Firstly, the tutorial3 target is installed to the BIN_INSTALL_DIR then the tutorial3ui.rc file that describes the layout of the user interface is installed to the application's data directory.
Make, Install And Run
만약 당신의 KDE4 설치 디렉토리에 대한 쓰기 권한을 가지지 않았다면, 당신의 홈 디렉토리에 설치할 수 있다.
- If you don't have write access to where your KDE4 installation directory, you can install it to a folder in your home directory.
CMake이 프로그램을 인스톨 하는 곳을 설정하기 위해서는, DCMAKE_INSTALL_PREFIX 스위치를 설정해야 한다. 아마 당신은 테스트를 위해 로컬 어디가에 그것을 설치하기를 원할 것이다.(당신의 KDE 디렉토리에 이 튜트리얼을 설치하는 것은 조금 바보스럽다.), 그러므로 정확히 다음과 실행하면 될 것이다. :
- To tell CMake where to install the program, set the DCMAKE_INSTALL_PREFIX switch. You probably just want to install it somewhere local for testing (it's probably a bit silly to go to the effort of installing these tutorials to your KDE directory), so the following might be appropriate:
mkdir build && cd build
cmake .. -DCMAKE_INSTALL_PREFIX=$HOME
make install
$HOME/bin/tutorial3
- which will create a KDE-like directory structure in your user's home directory directory and will install the executable to $HOME/bin/tutorial3.
나아가기
- 이제 saving and loading으로 진행할 수 있다.
- Now you can move on to saving and loading.