Development/Tutorials/Qt4 Ruby Tutorial/Chapter 11
Development/Tutorials/Qt4 Ruby Tutorial/Chapter 11
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Qt4 Ruby Tutorial |
Previous | Tutorial 10 - Smooth as Silk |
What's Next | Tutorial 12 - Hanging in the Air the Way Bricks Don't |
Further Reading | n/a |
Giving It a Shot
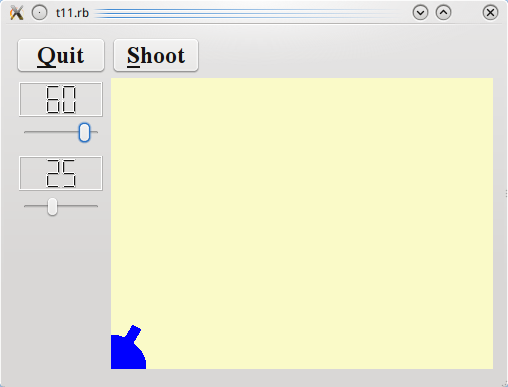
Files:
Overview
In this example we introduce a timer to implement animated shooting.
Line by Line Walkthrough
The CannonField now has shooting capabilities.
include Math
We include Math because we need the sin() and cos() functions.
@timerCount = 0
@autoShootTimer = Qt::Timer.new(self)
connect(@autoShootTimer, SIGNAL('timeout()'),
self, SLOT('moveShot()'))
@shootAngle = 0
@shootForce = 0
We initialize our new private variables and connect the Qt::Timer::timeout() signal to our moveShot() slot. We'll move the shot every time the timer times out.
The timerCount keeps track of the time passed since the shot was fired. The shootAngle is the cannon angle and shootForce is the cannon force when the shot was fired.
def shoot()
if @autoShootTimer.isActive()
return
end;
@timerCount = 0
@shootAngle = @currentAngle
@shootForce = @currentForce
@autoShootTimer.start(5)
end
This function shoots a shot unless a shot is in the air. The timerCount is reset to zero. The shootAngle and shootForce variables are set to the current cannon angle and force. Finally, we start the timer.
def moveShot()
region = Qt::Region.new(shotRect())
@timerCount += 1
shotR = shotRect()
if shotR.x() > width() || shotR.y() > height()
@autoShootTimer.stop()
else
region = region.unite(Qt::Region.new(shotR))
end
update(region)
end
moveShot() is the slot that moves the shot, called every 5 milliseconds when the Qt::Timer fires.
Its tasks are to compute the new position, update the screen with the shot in the new position, and if necessary, stop the timer.
First we make a Qt::Region that holds the old shotRect(). A Qt::Region is capable of holding any sort of region, and we'll use it here to simplify the painting. shotRect() returns the rectangle where the shot is now. It is explained in detail later.
Then we increment the timerCount, which has the effect of moving the shot one step along its trajectory.
Next we fetch the new shot rectangle.
If the shot has moved beyond the right or bottom edge of the widget we stop the timer, or we add the new shotRect() to the Qt::Region.
Finally, we repaint the Qt::Region. This will send a single paint event for just the one or two rectangles that need updating.
def paintEvent(event)
painter = Qt::Painter.new(self)
paintCannon(painter)
if @autoShootTimer.isActive()
paintShot(painter)
end
painter.end()
end
The paint event function has been simplified since the previous chapter. Most of the logic has been moved to the new paintShot() and paintCannon() functions.
def paintShot(painter)
painter.setPen(Qt::NoPen)
painter.setBrush(Qt::Brush.new(Qt::black))
painter.drawRect(shotRect())
end
This private function paints the shot by drawing a black filled rectangle.
We leave out the implementation of paintCannon(); it is the same as the Qt::Widget::paintEvent() reimplementation from the previous chapter.
def shotRect()
gravity = 4.0
time = @timerCount / 20.0
velocity = @shootForce
radians = @shootAngle * 3.14159265 / 180.0
velx = velocity * cos(radians)
vely = velocity * sin(radians)
x0 = (@barrelRect.right() + 5.0) * cos(radians)
y0 = (@barrelRect.right() + 5.0) * sin(radians)
x = x0 + velx * time
y = y0 + vely * time - 0.5 * gravity * time * time
result = Qt::Rect.new(0, 0, 6, 6)
result.moveCenter(Qt::Point.new(x.round, height() - 1 - y.round))
return result
end
This private function calculates the center point of the shot and returns the enclosing rectangle of the shot. It uses the initial cannon force and angle in addition to timerCount, which increases as time passes.
The formula used is the standard Newtonian formula for frictionless movement in a gravity field. For simplicity, we've chosen to disregard any Einsteinian effects.
We calculate the center point in a coordinate system where y coordinates increase upward. After we have calculated the center point, we construct a Qt::Rect with size 6 x 6 and move its center point to the point calculated above. In the same operation we convert the point into the widget's coordinate system (see The Coordinate System).
The only addition is the Shoot button.
shoot = Qt::PushButton.new(tr('&Shoot'))
shoot.setFont(Qt::Font.new('Times', 18, Qt::Font::Bold))
In the constructor we create and set up the Shoot button exactly like we did with the Quit button.
connect(shoot, SIGNAL('clicked()'), cannonField, SLOT('shoot()'))
Connects the clicked() signal of the Shoot button to the shoot() slot of the CannonField.
Running the Application
The cannon can shoot, but there's nothing to shoot at.
Exercises
Make the shot a filled circle. [Hint: Qt::Painter::drawEllipse() may help.]
Change the color of the cannon when a shot is in the air.