Development/Tutorials/Qt4 Ruby Tutorial/Chapter 07: Difference between revisions
Neverendingo (talk | contribs) m (Text replace - "<code ruby>" to "<syntaxhighlight lang="ruby">") |
No edit summary |
||
(5 intermediate revisions by 3 users not shown) | |||
Line 1: | Line 1: | ||
<languages /> | |||
name=One Thing Leads to Another| | {{<translate><!--T:1--> | ||
TutorialBrowser</translate>| | |||
pre=[[Development/Tutorials/Qt4_Ruby_Tutorial/Chapter_06|Tutorial 6 - Building Blocks Galore!]]| | series=[[Special:myLanguage/Development/Tutorials/Qt4_Ruby_Tutorial|<translate><!--T:2--> | ||
Qt4 Ruby Tutorial</translate>]]| | |||
next=[[Development/Tutorials/Qt4_Ruby_Tutorial/Chapter_08|Tutorial 8 - Preparing for Battle]] | name=<translate><!--T:3--> | ||
One Thing Leads to Another</translate>| | |||
pre=[[Special:myLanguage/Development/Tutorials/Qt4_Ruby_Tutorial/Chapter_06|<translate><!--T:4--> | |||
Tutorial 6 - Building Blocks Galore!</translate>]]| | |||
next=[[Special:myLanguage/Development/Tutorials/Qt4_Ruby_Tutorial/Chapter_08|<translate><!--T:5--> | |||
Tutorial 8 - Preparing for Battle</translate>]] | |||
}} | }} | ||
== One Thing Leads to Another == | <translate> | ||
== One Thing Leads to Another == <!--T:6--> | |||
</translate> | |||
<translate> | |||
<!--T:7--> | |||
[[Image:Qt4_Ruby_Tutorial_Screenshot_7.png|center]] | [[Image:Qt4_Ruby_Tutorial_Screenshot_7.png|center]] | ||
</translate> | |||
<translate> | |||
<!--T:8--> | |||
Files: | Files: | ||
</translate> | |||
* [http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/lcdrange.rb lcdrange.rb] | * [http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/lcdrange.rb lcdrange.rb] | ||
* [http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/t7.rb t7.rb] | * [http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/t7.rb t7.rb] | ||
=== Overview === | <translate> | ||
=== Overview === <!--T:9--> | |||
<!--T:10--> | |||
This example shows how to create custom widgets with signals and slots, and how to connect them together in more complex ways. For the first time, the source is split among several files. | This example shows how to create custom widgets with signals and slots, and how to connect them together in more complex ways. For the first time, the source is split among several files. | ||
</translate> | |||
=== Line by Line Walkthrough === | <translate> | ||
=== Line by Line Walkthrough === <!--T:11--> | |||
</translate> | |||
'''[http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/lcdrange.rb lcdrange.rb]''' | '''[http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/lcdrange.rb lcdrange.rb]''' | ||
This file is mainly lifted from Chapter 6; only the non-trivial changes are noted here. | <translate> | ||
<!--T:12--> | |||
This file is mainly lifted from [[Special:myLanguage/Development/Tutorials/Qt4_Ruby_Tutorial/Chapter_06|Chapter 6]]; only the non-trivial changes are noted here. | |||
</translate> | |||
<syntaxhighlight lang="ruby"> | <syntaxhighlight lang="ruby"> | ||
signals 'valueChanged(int)' | signals 'valueChanged(int)' | ||
slots 'setValue(int)' | slots 'setValue(int)' | ||
</ | </syntaxhighlight> | ||
<syntaxhighlight lang="ruby"> | <syntaxhighlight lang="ruby"> | ||
def value() | def value() | ||
Line 37: | Line 58: | ||
@slider.setValue(value) | @slider.setValue(value) | ||
end | end | ||
</ | </syntaxhighlight> | ||
<translate> | |||
<!--T:13--> | |||
These make up an interface between this widget and other components in a program. Until now, '''<tt>LCDRange</tt>''' didn't really have an API at all. | These make up an interface between this widget and other components in a program. Until now, '''<tt>LCDRange</tt>''' didn't really have an API at all. | ||
<!--T:14--> | |||
'''<tt>value()</tt>''' is a public function for accessing the value of the '''<tt>LCDRange</tt>''', '''<tt>setValue()</tt>''' is our first custom slot, and '''<tt>valueChanged()</tt>''' is our first custom signal. | '''<tt>value()</tt>''' is a public function for accessing the value of the '''<tt>LCDRange</tt>''', '''<tt>setValue()</tt>''' is our first custom slot, and '''<tt>valueChanged()</tt>''' is our first custom signal. | ||
<!--T:15--> | |||
Slots must be implemented in the normal way (a slot is also a Ruby member function). Signals are automatically implemented. Signals follow the access rules of protected Ruby functions (i.e., they can be emitted only by the class they are defined in or by classes inheriting from it). | Slots must be implemented in the normal way (a slot is also a Ruby member function). Signals are automatically implemented. Signals follow the access rules of protected Ruby functions (i.e., they can be emitted only by the class they are defined in or by classes inheriting from it). | ||
<!--T:16--> | |||
The '''<tt>valueChanged()</tt>''' signal is used when the '''<tt>LCDRange's</tt>''' value has changed. | The '''<tt>valueChanged()</tt>''' signal is used when the '''<tt>LCDRange's</tt>''' value has changed. | ||
<!--T:17--> | |||
The implementation of '''<tt>value()</tt>''' is straightforward. It simply returns the slider's value. | The implementation of '''<tt>value()</tt>''' is straightforward. It simply returns the slider's value. | ||
<!--T:18--> | |||
The implementation of '''<tt>setValue()</tt>''' is equally straightforward. Note that because the slider and LCD number are connected, setting the slider's value automatically updates the LCD number as well. In addition, the slider will automatically adjust the value if it is outside its legal range. | The implementation of '''<tt>setValue()</tt>''' is equally straightforward. Note that because the slider and LCD number are connected, setting the slider's value automatically updates the LCD number as well. In addition, the slider will automatically adjust the value if it is outside its legal range. | ||
</translate> | |||
<syntaxhighlight lang="ruby"> | <syntaxhighlight lang="ruby"> | ||
Line 56: | Line 85: | ||
connect(@slider, SIGNAL('valueChanged(int)'), | connect(@slider, SIGNAL('valueChanged(int)'), | ||
self, SIGNAL('valueChanged(int)')) | self, SIGNAL('valueChanged(int)')) | ||
</ | </syntaxhighlight> | ||
<translate> | |||
<!--T:19--> | |||
The first [http://doc.qt.nokia.com/latest/qobject.html#connect QObject::connect()] call is the same that you have seen in the previous chapter. The second is new; it connects slider's [http://doc.qt.nokia.com/latest/qabstractslider.html#valueChanged QAbstractSlider::valueChanged()] signal to this object's '''<tt>valueChanged()</tt>''' signal. Yes, that's right. Signals can be connected to other signals. When the first is emitted, the second signal is also emitted. | The first [http://doc.qt.nokia.com/latest/qobject.html#connect QObject::connect()] call is the same that you have seen in the previous chapter. The second is new; it connects slider's [http://doc.qt.nokia.com/latest/qabstractslider.html#valueChanged QAbstractSlider::valueChanged()] signal to this object's '''<tt>valueChanged()</tt>''' signal. Yes, that's right. Signals can be connected to other signals. When the first is emitted, the second signal is also emitted. | ||
<!--T:20--> | |||
Let's look at what happens when the user operates the slider. The slider sees that its value has changed and emits the [http://doc.qt.nokia.com/latest/qabstractslider.html#valueChanged QAbstractSlider::valueChanged()] signal. That signal is connected both to the [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] slot of the [http://doc.qt.nokia.com/latest/qlcdnumber.html Qt::LCDNumber] and to the '''<tt>valueChanged()</tt>''' signal of the '''<tt>LCDRange</tt>'''. | Let's look at what happens when the user operates the slider. The slider sees that its value has changed and emits the [http://doc.qt.nokia.com/latest/qabstractslider.html#valueChanged QAbstractSlider::valueChanged()] signal. That signal is connected both to the [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] slot of the [http://doc.qt.nokia.com/latest/qlcdnumber.html Qt::LCDNumber] and to the '''<tt>valueChanged()</tt>''' signal of the '''<tt>LCDRange</tt>'''. | ||
<!--T:21--> | |||
Thus, when the signal is emitted, '''<tt>LCDRange</tt>''' emits its own '''<tt>valueChanged()</tt>''' signal. In addition, [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] is called and shows the new number. | Thus, when the signal is emitted, '''<tt>LCDRange</tt>''' emits its own '''<tt>valueChanged()</tt>''' signal. In addition, [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] is called and shows the new number. | ||
<!--T:22--> | |||
Note that you're not guaranteed any particular order of execution; '''<tt>LCDRange::valueChanged()</tt>''' may be emitted before or after [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] is called. | Note that you're not guaranteed any particular order of execution; '''<tt>LCDRange::valueChanged()</tt>''' may be emitted before or after [http://doc.qt.nokia.com/latest/qlcdnumber.html#intValue-prop QLCDNumber::display()] is called. | ||
</translate> | |||
'''[http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/t7.rb t7.rb]''' | '''[http://www.darshancomputing.com/qt4-qtruby-tutorial/tutorial/t7/t7.rb t7.rb]''' | ||
Line 81: | Line 116: | ||
end | end | ||
end | end | ||
</ | </syntaxhighlight> | ||
<translate> | |||
<!--T:23--> | |||
When we create the nine '''<tt>LCDRange</tt>''' objects, we connect them using the [http://doc.qt.nokia.com/latest/signalsandslots.html signals and slots] mechanism. Each has its '''<tt>valueChanged()</tt>''' signal connected to the previous one's '''<tt>setValue()</tt>''' slot. Because '''<tt>LCDRange</tt>''' emits the '''<tt>valueChanged()</tt>''' signal when its value changes, we are here creating a chain of signals and slots. | When we create the nine '''<tt>LCDRange</tt>''' objects, we connect them using the [http://doc.qt.nokia.com/latest/signalsandslots.html signals and slots] mechanism. Each has its '''<tt>valueChanged()</tt>''' signal connected to the previous one's '''<tt>setValue()</tt>''' slot. Because '''<tt>LCDRange</tt>''' emits the '''<tt>valueChanged()</tt>''' signal when its value changes, we are here creating a chain of signals and slots. | ||
</translate> | |||
=== Running the Application === | <translate> | ||
=== Running the Application === <!--T:24--> | |||
<!--T:25--> | |||
On startup, the program's appearance is identical to the previous one. Try operating the slider to the bottom-right. | On startup, the program's appearance is identical to the previous one. Try operating the slider to the bottom-right. | ||
=== Exercises === | === Exercises === <!--T:26--> | ||
<!--T:27--> | |||
Use the bottom-right slider to set all LCDs to 50. Then set all but the last LCD to 40 by clicking once to the left of the bottom-middle slider handle. Now, use the bottom-left slider to set the first seven LCDs back to 50. | Use the bottom-right slider to set all LCDs to 50. Then set all but the last LCD to 40 by clicking once to the left of the bottom-middle slider handle. Now, use the bottom-left slider to set the first seven LCDs back to 50. | ||
<!--T:28--> | |||
Click to the left of the handle on the bottom-right slider. What happens? Why is this the correct behavior? | Click to the left of the handle on the bottom-right slider. What happens? Why is this the correct behavior? | ||
<!--T:29--> | |||
[[Category:Ruby]] | [[Category:Ruby]] | ||
</translate> |
Latest revision as of 14:19, 18 July 2012
Tutorial Series | Qt4 Ruby Tutorial |
Previous | Tutorial 6 - Building Blocks Galore! |
What's Next | Tutorial 8 - Preparing for Battle |
Further Reading | n/a |
One Thing Leads to Another
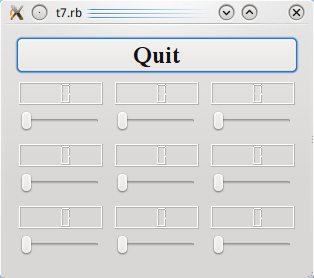
Files:
Overview
This example shows how to create custom widgets with signals and slots, and how to connect them together in more complex ways. For the first time, the source is split among several files.
Line by Line Walkthrough
This file is mainly lifted from Chapter 6; only the non-trivial changes are noted here.
signals 'valueChanged(int)'
slots 'setValue(int)'
def value()
@slider.value()
end
def setValue(value)
@slider.setValue(value)
end
These make up an interface between this widget and other components in a program. Until now, LCDRange didn't really have an API at all.
value() is a public function for accessing the value of the LCDRange, setValue() is our first custom slot, and valueChanged() is our first custom signal.
Slots must be implemented in the normal way (a slot is also a Ruby member function). Signals are automatically implemented. Signals follow the access rules of protected Ruby functions (i.e., they can be emitted only by the class they are defined in or by classes inheriting from it).
The valueChanged() signal is used when the LCDRange's value has changed.
The implementation of value() is straightforward. It simply returns the slider's value.
The implementation of setValue() is equally straightforward. Note that because the slider and LCD number are connected, setting the slider's value automatically updates the LCD number as well. In addition, the slider will automatically adjust the value if it is outside its legal range.
connect(@slider, SIGNAL('valueChanged(int)'),
lcd, SLOT('display(int)'))
connect(@slider, SIGNAL('valueChanged(int)'),
self, SIGNAL('valueChanged(int)'))
The first QObject::connect() call is the same that you have seen in the previous chapter. The second is new; it connects slider's QAbstractSlider::valueChanged() signal to this object's valueChanged() signal. Yes, that's right. Signals can be connected to other signals. When the first is emitted, the second signal is also emitted.
Let's look at what happens when the user operates the slider. The slider sees that its value has changed and emits the QAbstractSlider::valueChanged() signal. That signal is connected both to the QLCDNumber::display() slot of the Qt::LCDNumber and to the valueChanged() signal of the LCDRange.
Thus, when the signal is emitted, LCDRange emits its own valueChanged() signal. In addition, QLCDNumber::display() is called and shows the new number.
Note that you're not guaranteed any particular order of execution; LCDRange::valueChanged() may be emitted before or after QLCDNumber::display() is called.
previousRange = nil
for row in 0..2
for column in 0..2
lcdRange = LCDRange.new()
grid.addWidget(lcdRange, row, column)
unless previousRange.nil?
connect(lcdRange, SIGNAL('valueChanged(int)'),
previousRange, SLOT('setValue(int)'))
end
previousRange = lcdRange
end
end
When we create the nine LCDRange objects, we connect them using the signals and slots mechanism. Each has its valueChanged() signal connected to the previous one's setValue() slot. Because LCDRange emits the valueChanged() signal when its value changes, we are here creating a chain of signals and slots.
Running the Application
On startup, the program's appearance is identical to the previous one. Try operating the slider to the bottom-right.
Exercises
Use the bottom-right slider to set all LCDs to 50. Then set all but the last LCD to 40 by clicking once to the left of the bottom-middle slider handle. Now, use the bottom-left slider to set the first seven LCDs back to 50.
Click to the left of the handle on the bottom-right slider. What happens? Why is this the correct behavior?