Development/Tutorials/KCmdLineArgs: Difference between revisions
m (Chage Language Navigation Bar incorrected (from 'Saving_and_loading' to 'KCmdLineArgs')) |
(→tutorial4ui.rc: change the number to 5 ;)) |
||
Line 207: | Line 207: | ||
</code> | </code> | ||
=== | ===tutorial5ui.rc=== | ||
<code xml n> | <code xml n> | ||
<?xml version="1.0" encoding="UTF-8"?> | <?xml version="1.0" encoding="UTF-8"?> |
Revision as of 12:59, 12 February 2008
Development/Tutorials/KCmdLineArgs
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Beginner Tutorial |
Previous | Tutorial 4 - Loading and saving |
What's Next | Tutorial 6 - ### (TODO User:milliams) |
Further Reading | KCmdLineArgs KCmdLineOptions |
Abstract
Now that we have a text editor which can open and save files. We will now make the editor act more like a desktop application by enabling it to open files from command line arguments or even using Open with from within Dolphin.
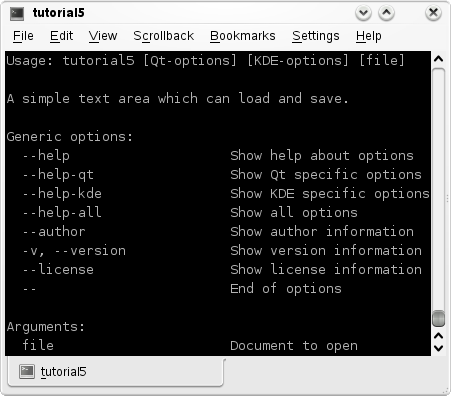
The Code
main.cpp
- include <KApplication>
- include <KAboutData>
- include <KCmdLineArgs>
- include <KUrl> //new
- include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial5", "tutorial5",
ki18n("Tutorial 5"), "1.0",
ki18n("A simple text area which can load and save."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KCmdLineOptions options; //new
options.add("+[file]", ki18n("Document to open")); //new
KCmdLineArgs::addCmdLineOptions(options); //new
KApplication app;
MainWindow* window = new MainWindow();
window->show();
KCmdLineArgs *args = KCmdLineArgs::parsedArgs(); //new
if(args->count()) //new
{
window->openFile(args->url(0).url()); //new
}
return app.exec();
}
mainwindow.h
- ifndef MAINWINDOW_H
- define MAINWINDOW_H
- include <KXmlGuiWindow>
- include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent=0);
void openFile(const QString &inputFileName); //new
private:
KTextEdit* textArea;
void setupActions();
QString fileName;
private slots:
void newFile();
void openFile();
void saveFile();
void saveFileAs();
void saveFileAs(const QString &outputFileName);
};
- endif
mainwindow.cpp
- include "mainwindow.h"
- include <KApplication>
- include <KAction>
- include <KLocale>
- include <KActionCollection>
- include <KStandardAction>
- include <KFileDialog>
- include <KMessageBox>
- include <KIO/NetAccess>
- include <KSaveFile>
- include <QTextStream>
MainWindow::MainWindow(QWidget *parent)
: KXmlGuiWindow(parent),
fileName(QString())
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KAction* clearAction = new KAction(this);
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("document-new"));
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
actionCollection()->addAction("clear", clearAction);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()),
actionCollection());
KStandardAction::open(this, SLOT(openFile()),
actionCollection());
KStandardAction::save(this, SLOT(saveFile()),
actionCollection());
KStandardAction::saveAs(this, SLOT(saveFileAs()),
actionCollection());
KStandardAction::openNew(this, SLOT(newFile()),
actionCollection());
setupGUI();
}
void MainWindow::newFile()
{
fileName.clear();
textArea->clear();
}
void MainWindow::saveFileAs(const QString &outputFileName)
{
KSaveFile file(outputFileName);
file.open();
QByteArray outputByteArray;
outputByteArray.append(textArea->toPlainText());
file.write(outputByteArray);
file.finalize();
file.close();
fileName = outputFileName;
}
void MainWindow::saveFileAs()
{
saveFileAs(KFileDialog::getSaveFileName());
}
void MainWindow::saveFile()
{
if(!fileName.isEmpty())
{
saveFileAs(fileName);
}
else
{
saveFileAs();
}
}
void MainWindow::openFile() //changed
{
openFile(KFileDialog::getOpenFileName());
}
void MainWindow::openFile(const QString &inputFileName) //new
{
QString tmpFile;
if(KIO::NetAccess::download(inputFileName, tmpFile,
this))
{
QFile file(tmpFile);
file.open(QIODevice::ReadOnly);
textArea->setPlainText(QTextStream(&file).readAll());
fileName = inputFileName;
KIO::NetAccess::removeTempFile(tmpFile);
}
else
{
KMessageBox::error(this,
KIO::NetAccess::lastErrorString());
}
}
tutorial5ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE kpartgui SYSTEM "kpartgui.dtd">
<gui name="tutorial5" version="1">
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
<MenuBar>
<Menu name="file" >
<Action name="clear" />
</Menu>
</MenuBar>
</gui>
This is identical to the tutorialxui.rc from the last two tutorials except tutorialx is now tutorial5.
Explanation
mainwindow.h
Here we have done nothing but add a new openFile function which takes a QString
void openFile(const QString &inputFileName);
mainwindow.cpp
There's no new code here, only rearranging. Everything from void openFile() has been moved into void openFile(const QString &inputFileName) except the call to KFileDialog::getOpenFileName().
This way, we can call openFile() if we want to display a dialog, or we can call openFile(QString) if we know the name of the file already.
main.cpp
This is where all the KCmdLineArgs magic happens.
Make, Install And Run
CMakeLists.txt
project(tutorial5)
find_package(KDE4 REQUIRED)
include_directories( ${KDE4_INCLUDES} )
set(tutorial5_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial5 ${tutorial5_SRCS})
target_link_libraries(tutorial5 ${KDE4_KDEUI_LIBS}
${KDE4_KIO_LIBS})
install(TARGETS tutorial5 DESTINATION ${BIN_INSTALL_DIR})
install( FILES tutorial5ui.rc
DESTINATION ${DATA_INSTALL_DIR}/tutorial5 )
With this file, the tutorial can built and run in the same way as tutorial 3 and 4. For more information, see tutorial 3.
mkdir build && cd build cmake .. -DCMAKE_INSTALL_PREFIX=$HOME make install $HOME/bin/tutorial5
Moving On
Now you can move on to the ### (TODO User:milliams) tutorial.