Marble/Runners/MarblePythonLoadingOSM
Tutorial Series | Marble Python Tutorial |
Previous | Tutorial 4 - Loading KML files into Marble |
What's Next | Tutorial 6 - Displaying a GeoData Placemark |
Further Reading | n/a |
Exporting OSM
Osm files can be used for creating small street maps or can be scaled to store streets worldwide. OSM files can be exported from OpenStreetMaps by clicking the export button as shown below.
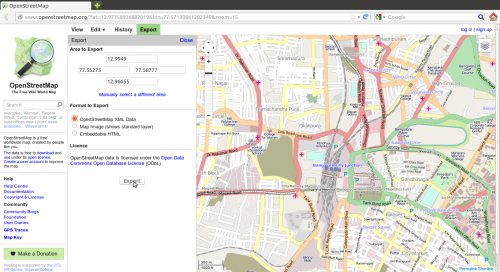
Loading OSM files into a Marble Widget

Marble uses so-called runners to calculate routes, do reverse geocoding, parse files and search for placemarks (cities, addresses, points of interest, ...). This tutorial shows how to open a .osm file and display it into the Marble Widget.
#!/usr/bin/env python
from PyQt4.QtCore import *
from PyQt4.QtGui import *
from PyKDE4.marble import *
import sys
import os
def main():
app = QApplication(sys.argv)
# check the file was loaded
if(len(sys.argv) < 2):
print("Usage: python " + sys.argv[0] + " file.osm")
return 1
# load file
inputFile = os.path.abspath(sys.argv[-1])
print(inputFile)
# create the marble widget
marble = Marble.MarbleWidget()
# resize the widget and add a window title
marble.resize(800, 600)
marble.setWindowTitle("my_marble")
# Load the OpenStreetMap map
marble.setMapThemeId("earth/openstreetmap/openstreetmap.dgml")
# center on Bucharest and Zoom
marble.centerOn(Marble.GeoDataCoordinates(77.5674, 12.9782, 0, Marble.GeoDataCoordinates.Degree));
marble.setZoom(3000)
# add the kml file to the model
marble.model().addGeoDataFile(inputFile)
# add the widget to the KMainWindow
marble.show()
# run the app
app.exec_()
main()
Copy and paste the code above into a text editor. Then save it as loading_osm.py and run it with:
python loading_osm.py some-file.osm
You should get a Marble Widget which displays your OSM file. For example, download and unpack Map.osm (a LinearRing representing Bangalore city junction), place it in the same folder as your loading_osm.py file and run python loading_osm.py map.osm. The result should be similar to this (note the map may reset the zoom level when it adds the .osm file data):
The placemarks on the map are clickable and trigger a dialog. The screenshot below is of the dialog of Bangalore City Station triggered by clicking it on the map.
