Languages/Python/PyKDE WebKit Tutorial
Creating a simple web browser application in PyKDE using the WebKit widget from Qt.
Python is a powerful yet intuitive programming language. PyQt and PyKDE are bindings which let you write GUI programmes using Qt and KDE widgets in Python.
PyKDE is used in KDE for the printer-applet tool from kdeutils which shows current print jobs, system-config-printer-kde which edits printer settings and for Guidance Power Manager a battery applet. PyKDE is used extensively in Kubuntu, a KDE based Linux distribution. It is used to write the installer (Ubiquity), packaging tools such as software-properties and the tool used for distribution upgrades.
Here we will create a simple web browser application. We start with a basic Qt version of our application.
#!/usr/bin/env python
import sys
from PyQt4.QtCore import *
from PyQt4.QtGui import *
from PyQt4.QtWebKit import *
app = QApplication(sys.argv)
web = QWebView()
web.load(QUrl("http://kubuntu.org"))
web.show()
sys.exit(app.exec_())
Write this out in a file and save it as webkit1.py. Python applications are not compiled, you can run this from a command line with python webkit1.py (or you can set it as executable and just run ./webkit1.py.
The first line of the code tells Linux to run the file with Python.
The next block imports some Python program libraries. sys contains useful functions to do with the system. The PyQt libraries contain GUI widgets and related code.
All Qt applications need to create a QApplication object. Our code here creates the object passing it sys.argv which contains and command line options used to start the application (none in our case) and saves it to a variable called app.
Next we create a QWebView object. QWebView is the widget from Qt which gives us a WebKit based browser. The QWebView documentation shows us methods which we can call on our object, the one we are interested in is load(QUrl) which loads a web page. So we call that in the next line. Then we use show() to display the widget, it has no parent widget so it will be displayed as a window on your desktop.
Finally we start the application with app.exec_(). In C++ this method is called just exec() but we need to add an underscore in Python because exec is a reserved word. exec_() will finish when you close the window, at which point it will return 0 (or another exit code if there are problems). This gets passed to Python's sys.exit() function which will quit the programme.
It will look like this
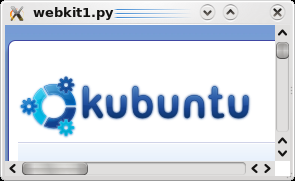