Archive:Development/Tutorials/KCmdLineArgs (zh CN)
Appearance
Template:TutorialBrowser (zh CN)
摘要
我们要有一个文字编辑器,可以用来加载保存文件。我们要让这个编辑器象应用程序一样,用命令行参数来打开文件,甚至在Dolphin里用"以...打开"的方式来打开文件。
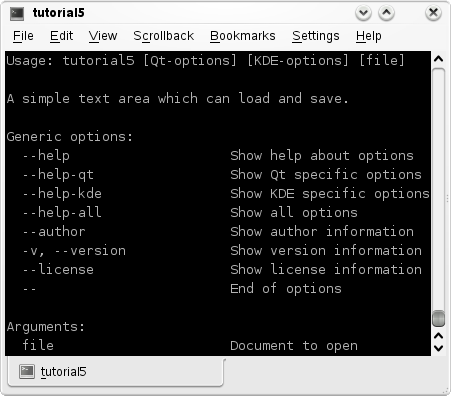
代码
main.cpp
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
#include <KUrl> //新加
#include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial5", "tutorial5",
ki18n("Tutorial 5"), "1.0",
ki18n("A simple text area which can load and save."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KCmdLineOptions options; //新加
options.add("+[file]", ki18n("Document to open")); //新加
KCmdLineArgs::addCmdLineOptions(options); //新加
KApplication app;
MainWindow* window = new MainWindow();
window->show();
KCmdLineArgs *args = KCmdLineArgs::parsedArgs(); //新加
if(args->count()) //新加
{
window->openFile(args->url(0).url()); //新加
}
return app.exec();
}
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <KXmlGuiWindow>
#include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent=0);
void openFile(const QString &inputFileName); //新加
private:
KTextEdit* textArea;
void setupActions();
QString fileName;
private slots:
void newFile();
void openFile();
void saveFile();
void saveFileAs();
void saveFileAs(const QString &outputFileName);
};
#endif
mainwindow.cpp
#include "mainwindow.h"
#include <KApplication>
#include <KAction>
#include <KLocale>
#include <KActionCollection>
#include <KStandardAction>
#include <KFileDialog>
#include <KMessageBox>
#include <KIO/NetAccess>
#include <KSaveFile>
#include <QTextStream>
MainWindow::MainWindow(QWidget *parent)
: KXmlGuiWindow(parent),
fileName(QString())
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KAction* clearAction = new KAction(this);
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("document-new"));
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
actionCollection()->addAction("clear", clearAction);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()),
actionCollection());
KStandardAction::open(this, SLOT(openFile()),
actionCollection());
KStandardAction::save(this, SLOT(saveFile()),
actionCollection());
KStandardAction::saveAs(this, SLOT(saveFileAs()),
actionCollection());
KStandardAction::openNew(this, SLOT(newFile()),
actionCollection());
setupGUI();
}
void MainWindow::newFile()
{
fileName.clear();
textArea->clear();
}
void MainWindow::saveFileAs(const QString &outputFileName)
{
KSaveFile file(outputFileName);
file.open();
QByteArray outputByteArray;
outputByteArray.append(textArea->toPlainText());
file.write(outputByteArray);
file.finalize();
file.close();
fileName = outputFileName;
}
void MainWindow::saveFileAs()
{
saveFileAs(KFileDialog::getSaveFileName());
}
void MainWindow::saveFile()
{
if(!fileName.isEmpty())
{
saveFileAs(fileName);
}
else
{
saveFileAs();
}
}
void MainWindow::openFile() //变更
{
openFile(KFileDialog::getOpenFileName());
}
void MainWindow::openFile(const QString &inputFileName) //新加
{
QString tmpFile;
if(KIO::NetAccess::download(inputFileName, tmpFile,
this))
{
QFile file(tmpFile);
file.open(QIODevice::ReadOnly);
textArea->setPlainText(QTextStream(&file).readAll());
fileName = inputFileName;
KIO::NetAccess::removeTempFile(tmpFile);
}
else
{
KMessageBox::error(this,
KIO::NetAccess::lastErrorString());
}
}
tutorial5ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<gui name="tutorial5"
version="1"
xmlns="http://www.kde.org/standards/kxmlgui/1.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.kde.org/standards/kxmlgui/1.0
http://www.kde.org/standards/kxmlgui/1.0/kxmlgui.xsd" >
<MenuBar>
<Menu name="file" >
<Action name="clear" />
</Menu>
</MenuBar>
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
</gui>
这和教程x的ui.rc一样,唯一改掉的就是从教程x现在变成了教程五.
注解
mainwindow.h
这里我们就加了个openFile,它接受一个QString作为参数。
void openFile(const QString &inputFileName);
mainwindow.cpp
没有什么新东西,重新整理一下而已。所有void openFile()里的东西都被移到了void openFile(const QString &inputFileName)里,除了KFileDialog::getOpenFileName()的调用。
这样,如果我们要一个对话框就调用openFile(),如果指导文件名,调用openFile(QString)就可以了。
main.cpp
这里就是KCmdLineArgs产生神奇效应的地方。
构建,安装,运行
CMakeLists.txt
project(tutorial5)
find_package(KDE4 REQUIRED)
include_directories( ${KDE4_INCLUDES} )
set(tutorial5_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial5 ${tutorial5_SRCS})
target_link_libraries(tutorial5 ${KDE4_KDEUI_LIBS}
${KDE4_KIO_LIBS})
install(TARGETS tutorial5 DESTINATION ${BIN_INSTALL_DIR})
install( FILES tutorial5ui.rc
DESTINATION ${DATA_INSTALL_DIR}/tutorial5 )
有了这个文件,这个教程就可以象教程三教程四里的一样运行了。更多信息,详见教程三。
mkdir build && cd build cmake .. -DCMAKE_INSTALL_PREFIX=$HOME make install $HOME/bin/tutorial5
继续
现在你可以继续了 ### (TODO User:milliams)。