Development/Tutorials/Programming Tutorial KDE 4/Using KConfig
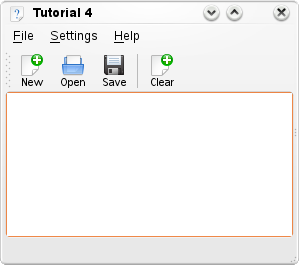
Abstract
Author: Fabian Korak
Author: Matt Williams(presets)
In this tutorial, we're going to be showing you how to utilise KConfig XT in an application. It is recommended that you read Development/Tutorials/Using KConfig XT before continuing in order to familiarise yourself with the framework.
Prerequisites
KConfig XT
At first we make new File called tutorial4.kcfg within the source directory of your project.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE kcfg SYSTEM "http://www.kde.org/standards/kcfg/1.0/kcfg.xsd">
<kcfg>
<kcfgfile name="kjotsrc"/>
<include>kglobalsettings.h</include>
<group name="kjots">
<entry name="showAction" type="Bool">
<label>Whether setupAction is called</label>
<default>true</default>
</entry>
</group>
</kcfg>
This code makes a setting of the type bool, which determines whether we want to do something. Then we set the default value to be true.
Always start the value of <entry name=""> in lower case since KConfig XT sets them to lower case within the generated headers either way. If you don't this could give you some nearly untraceable errors
Now we make a new File called tutorial4.kcfgc.
File=tutorial4.kcfg
ClassName=tutorial4
Singleton=true
Mutators=true
This should set up your configuration for now.
The Code
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <KXmlGuiWindow>
#include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
void setupActions();
void setupConfig();
void readConfig();
void saveSettings();
int m_showAction;
};
#endif
There are some new voids in here, as well as m_showAction witch determines the value stored in KConfig XT. Despite that value being a bool we can actually use an integer here, since the type is converted either way.
mainwindow.cpp
#include "mainwindow.h"
#include "tutorial4.h"
#include <KApplication>
#include <KAction>
#include <KLocale>
#include <KActionCollection>
#include <KStandardAction>
#include <KConfigDialog>
MainWindow::MainWindow(QWidget *parent) : KXmlGuiWindow(parent)
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupConfig();
}
void MainWindow::setupActions()
{
QAction* clearAction = actionCollection()->addAction( "clear" );
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("filenew"));
clearAction->setShortcut(Qt::CTRL+ Qt::Key_W);
connect(clearAction, SIGNAL(triggered(bool)), textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
setupGUI();
}
void MainWindow::setupConfig()
{
readConfig();
}
void MainWindow::readConfig() {
m_showAction = tutorial4::showAction();
if(m_showAction = (true))
setupActions();
}
void MainWindow::saveSettings() {
tutorial4::setShowAction(m_showAction);
tutorial4::self()->writeConfig();
}
At first we import "tutorial4.h", which should be auto-generated at runtime by KConfig XT every time you compile this. MainWindow and SetupActions are copied from the tutorials before. The only thing new is that we now call a new void called setupConfig. This then calls readConfig. We could also call saveSettings(but only after we called readConfig), but since we don't change any values there this is more of a "proof of concept".
Within readConfig we assign m_showAction the value of the setting showAction. If this returns true setupsActions makes buttons and all that. When false it doesn't.
The setShowActions() in saveSettings() overwrites showAction with a value of your choice, in this case with itself. Then you need to call writeConfig() to apply the changes you made.
main.cpp
#include "mainwindow.h"
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial4", "tutorial4",
"0.4", "KMessageBox popup",
KAboutData::License_GPL, "(c) 2007" );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
No news in here
tutorial4ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<gui name="tutorial4"
version="1"
xmlns="http://www.kde.org/standards/kxmlgui/1.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.kde.org/standards/kxmlgui/1.0
http://www.kde.org/standards/kxmlgui/1.0/kxmlgui.xsd" >
<MenuBar>
<Menu name="file" >
<text>&File</text>
<Action name="clear" />
</Menu>
</MenuBar>
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
</gui>
CMake
CMakeLists.txt
PROJECT(tutorial4)
FIND_PACKAGE(KDE4 REQUIRED)
INCLUDE_DIRECTORIES( ${KDE4_INCLUDES} ${CMAKE_CURRENT_BINARY_DIR})
SET(tutorial4_SRCS
main.cpp
mainwindow.cpp
)
KDE4_ADD_KCFG_FILES(tutorial4_SRCS settings.kcfgc)
KDE4_ADD_EXECUTABLE(tutorial4 ${tutorial4_SRCS})
TARGET_LINK_LIBRARIES( tutorial4 ${KDE4_KDEUI_LIBS})
install( TARGETS tutorial4 DESTINATION ${BIN_INSTALL_DIR})
install( FILES tutorial4ui.rc DESTINATION ${DATA_INSTALL_DIR}/tutorial4)
install( FILES tutorial4.kcfg DESTINATION ${KCFG_INSTALL_DIR} )
Moving On
For a better understanding of the XML used in KConfig XT you could try to write an Xml Parser