Development/Tutorials/Using KXmlGuiWindow: Difference between revisions
m (→Kompile it: Spelling) |
No edit summary |
||
(35 intermediate revisions by 11 users not shown) | |||
Line 1: | Line 1: | ||
{{TutorialBrowser| | {{TutorialBrowser| | ||
series=Beginner Tutorial| | series=Beginner Tutorial| | ||
name=How To Use | name=How To Use KXmlGuiWindow| | ||
pre=[[Development/Tutorials | pre=[[Development/Tutorials/First_program|Tutorial 1 - Hello World]]| | ||
next=[[Development/Tutorials | next=[[Development/Tutorials/Using_KActions|Tutorial 3 - KActions and XMLGUI]]| | ||
reading={{class| | reading={{class|KXmlGuiWindow}} | ||
}} | }} | ||
==Abstract== | ==Abstract== | ||
This tutorial carries on from [[Development/Tutorials | This tutorial carries on from [[Development/Tutorials/First_program|First Program Tutorial]] and will introduce the {{class|KXmlGuiWindow}} class. | ||
In the previous tutorial, the program caused a dialog box to pop up but we're going to take steps towards a functioning application. | In the previous tutorial, the program caused a dialog box to pop up but we're going to take steps towards a functioning application. | ||
Line 19: | Line 20: | ||
[[image:introtokdetutorial2.png|frame|center]] | [[image:introtokdetutorial2.png|frame|center]] | ||
== | ==KXmlGuiWindow== | ||
In order to have a useful KXmlGuiWindow, we must subclass it. So we create two files, a <tt>mainwindow.cpp</tt> and a <tt>mainwindow.h</tt> which will contain our code | {{class|KXmlGuiWindow}} provides a full main window view with menubars, toolbars, a statusbar and a main area in the centre for a large widget. For example the help-menu is predefined. Most KDE applications will derive from this class as it provides an easy way to define menu and toolbar layouts through XML files (this technology is called XMLGUI). While we will not be using XMLGUI in ''this'' tutorial, we will use it in the next. | ||
In order to have a useful KXmlGuiWindow, we must subclass it. So we create two files, a <tt>mainwindow.cpp</tt> and a <tt>mainwindow.h</tt> which will contain our code. | |||
===mainwindow.h=== | ===mainwindow.h=== | ||
< | <syntaxhighlight lang="cpp-qt"> | ||
#ifndef MAINWINDOW_H | #ifndef MAINWINDOW_H | ||
#define MAINWINDOW_H | #define MAINWINDOW_H | ||
Line 41: | Line 44: | ||
#endif | #endif | ||
</ | </syntaxhighlight> | ||
First we Subclass KXmlGuiWindow on line 7 | First we Subclass KXmlGuiWindow on line 7 with <tt>class MainWindow : public KXmlGuiWindow</tt>. | ||
Then we declare the constructor with <tt>MainWindow(QWidget *parent=0);</tt>. | Then we declare the constructor with <tt>MainWindow(QWidget *parent=0);</tt>. | ||
Line 49: | Line 52: | ||
===mainwindow.cpp=== | ===mainwindow.cpp=== | ||
< | <syntaxhighlight lang="cpp-qt"> | ||
#include "mainwindow.h" | #include "mainwindow.h" | ||
MainWindow::MainWindow(QWidget *parent) : KXmlGuiWindow(parent) | MainWindow::MainWindow(QWidget *parent) : KXmlGuiWindow(parent) | ||
{ | { | ||
textArea = new KTextEdit; | textArea = new KTextEdit(); | ||
setCentralWidget(textArea); | setCentralWidget(textArea); | ||
setupGUI(); | setupGUI(); | ||
} | } | ||
</ | </syntaxhighlight> | ||
First, of course, on line 1 we have to include the header file containing the class declaration. | First, of course, on line 1 we have to include the header file containing the class declaration. | ||
On line 5, we initialise our text editor with an object. Then on line 6 we use | On line 5, we initialise our text editor with an object. Then on line 6 we use KXmlGuiWindow's built-in setCentralWidget() function which tells the KXmlGuiWindow what should appear in the central section of the window. | ||
Finally, KXmlGuiWindow::setupGUI() is called which does a lot of behind-the-scenes stuff and creates the default menu bars (Settings, Help). | Finally, KXmlGuiWindow::setupGUI() is called which does a lot of behind-the-scenes stuff and creates the default menu bars (Settings, Help). | ||
Line 68: | Line 71: | ||
In order to actually run this window, we need to add a few lines in main.cpp: | In order to actually run this window, we need to add a few lines in main.cpp: | ||
===main.cpp=== | ===main.cpp=== | ||
< | <syntaxhighlight lang="cpp-qt"> | ||
#include <KApplication> | #include <KApplication> | ||
#include <KAboutData> | #include <KAboutData> | ||
#include <KCmdLineArgs> | #include <KCmdLineArgs> | ||
#include <KLocale> | |||
#include "mainwindow.h" | #include "mainwindow.h" | ||
Line 77: | Line 81: | ||
int main (int argc, char *argv[]) | int main (int argc, char *argv[]) | ||
{ | { | ||
KAboutData aboutData( "tutorial2", | KAboutData aboutData( "tutorial2", 0, | ||
" | ki18n("Tutorial 2"), "1.0", | ||
KAboutData::License_GPL, "(c) | ki18n("A simple text area"), | ||
KAboutData::License_GPL, | |||
ki18n("Copyright (c) 2007 Developer") ); | |||
KCmdLineArgs::init( argc, argv, &aboutData ); | KCmdLineArgs::init( argc, argv, &aboutData ); | ||
Line 89: | Line 95: | ||
return app.exec(); | return app.exec(); | ||
} | } | ||
</ | </syntaxhighlight> | ||
The only new lines here (compared to Tutorial 1) are | The only new lines here (compared to Tutorial 1) are 6, 19 and 20. On line 19, we create our MainWindow object and then on line 20, we display it. | ||
==CMake== | ==CMake== | ||
The best way to build the program is to use CMake. All that's changed since tutorial 1 is that <tt>mainwindow.cpp</tt> has been added to the sources list and any <tt>tutorial1</tt> has become <tt>tutorial2</tt>. | The best way to build the program is to use CMake. All that's changed since tutorial 1 is that <tt>mainwindow.cpp</tt> has been added to the sources list and any <tt>tutorial1</tt> has become <tt>tutorial2</tt>. | ||
===CMakeLists.txt=== | ===CMakeLists.txt=== | ||
< | <syntaxhighlight lang="cmake"> | ||
project (tutorial2) | project (tutorial2) | ||
find_package(KDE4 REQUIRED) | find_package(KDE4 REQUIRED) | ||
include_directories( ${KDE4_INCLUDES} ) | include_directories(${KDE4_INCLUDES}) | ||
set(tutorial2_SRCS | set(tutorial2_SRCS | ||
main.cpp | |||
mainwindow.cpp | |||
) | ) | ||
kde4_add_executable(tutorial2 ${tutorial2_SRCS}) | kde4_add_executable(tutorial2 ${tutorial2_SRCS}) | ||
target_link_libraries( tutorial2 ${KDE4_KDEUI_LIBS}) | target_link_libraries(tutorial2 ${KDE4_KDEUI_LIBS}) | ||
</ | </syntaxhighlight> | ||
===Compile | === Compile and run === | ||
To compile, link and run it, | To compile, link and run it, make sure you have [[Getting_Started/Build|set up a correct build environment]] and issue: | ||
<syntaxhighlight lang="bash"> | |||
cmake . && make && ./tutorial2 | |||
</syntaxhighlight> | |||
==Moving On== | ==Moving On== | ||
Now you can move on to [[Development/Tutorials | Now you can move on to [[Development/Tutorials/Using_KActions|using KActions]]. | ||
[[Category:C++]] | [[Category:C++]] |
Revision as of 12:53, 13 July 2012
Tutorial Series | Beginner Tutorial |
Previous | Tutorial 1 - Hello World |
What's Next | Tutorial 3 - KActions and XMLGUI |
Further Reading | KXmlGuiWindow |
Abstract
This tutorial carries on from First Program Tutorial and will introduce the KXmlGuiWindow class.
In the previous tutorial, the program caused a dialog box to pop up but we're going to take steps towards a functioning application.
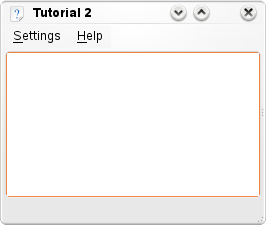
KXmlGuiWindow
KXmlGuiWindow provides a full main window view with menubars, toolbars, a statusbar and a main area in the centre for a large widget. For example the help-menu is predefined. Most KDE applications will derive from this class as it provides an easy way to define menu and toolbar layouts through XML files (this technology is called XMLGUI). While we will not be using XMLGUI in this tutorial, we will use it in the next.
In order to have a useful KXmlGuiWindow, we must subclass it. So we create two files, a mainwindow.cpp and a mainwindow.h which will contain our code.
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <KXmlGuiWindow>
#include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
};
#endif
First we Subclass KXmlGuiWindow on line 7 with class MainWindow : public KXmlGuiWindow.
Then we declare the constructor with MainWindow(QWidget *parent=0);.
And finally we declare a pointer to the object that will make up the bulk of our program. KTextEdit is a generic richtext editor with some KDE niceties like cursor auto-hiding.
mainwindow.cpp
#include "mainwindow.h"
MainWindow::MainWindow(QWidget *parent) : KXmlGuiWindow(parent)
{
textArea = new KTextEdit();
setCentralWidget(textArea);
setupGUI();
}
First, of course, on line 1 we have to include the header file containing the class declaration.
On line 5, we initialise our text editor with an object. Then on line 6 we use KXmlGuiWindow's built-in setCentralWidget() function which tells the KXmlGuiWindow what should appear in the central section of the window.
Finally, KXmlGuiWindow::setupGUI() is called which does a lot of behind-the-scenes stuff and creates the default menu bars (Settings, Help).
Back to main.cpp
In order to actually run this window, we need to add a few lines in main.cpp:
main.cpp
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
#include <KLocale>
#include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial2", 0,
ki18n("Tutorial 2"), "1.0",
ki18n("A simple text area"),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
The only new lines here (compared to Tutorial 1) are 6, 19 and 20. On line 19, we create our MainWindow object and then on line 20, we display it.
CMake
The best way to build the program is to use CMake. All that's changed since tutorial 1 is that mainwindow.cpp has been added to the sources list and any tutorial1 has become tutorial2.
CMakeLists.txt
project (tutorial2)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(tutorial2_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial2 ${tutorial2_SRCS})
target_link_libraries(tutorial2 ${KDE4_KDEUI_LIBS})
Compile and run
To compile, link and run it, make sure you have set up a correct build environment and issue:
cmake . && make && ./tutorial2
Moving On
Now you can move on to using KActions.