Development/Tutorials/Using KActions
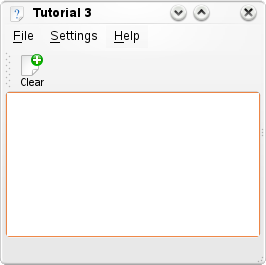
Abstract
We're going to introduce the concept of actions. Actions are a unified way of supplying the user with ways to interact with your program. Say, for example, we want to let the user clear the text box by clicking a button in the toolbar, from an option in the File menu or through a keyboard shortcut; we can provide all of those through one KAction.
Prerequisites
KAction
Creating Your Own
KStandardAction
The Code
mainwindow.h
- ifndef MAINWINDOW_H
- define MAINWINDOW_H
- include <KMainWindow>
- include <KTextEdit>
class MainWindow : public KMainWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
void setupActions();
};
- endif
mainwindow.cpp
- include "mainwindow.h"
- include <KApplication>
- include <KAction>
- include <KStandardAction>
MainWindow::MainWindow(QWidget *parent) : KMainWindow(parent)
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
KAction* clearAction = new KAction(actionCollection(), "clear");
clearAction->setText("Clear");
clearAction->setIcon(KIcon("filenew"));
clearAction->setShortcut(Qt::CTRL+Qt::Key_W);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
setupGUI();
}
main.cpp
- include <KApplication>
- include <KAboutData>
- include <KCmdLineArgs>
- include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial3", "Tutorial 3",
"1.0", "A simple text area using KAction etc.", KAboutData::License_GPL,
"(c) 2006" );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
XMLGUI
tutorial3ui.rc
<!DOCTYPE kpartgui SYSTEM "kpartgui.dtd">
<kpartgui name="tutorial3" version="1">
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
<MenuBar>
<Menu name="file" >
<text>&File</text>
<Action name="clear" />
</Menu>
</MenuBar>
</kpartgui>
CMake
PROJECT(tutorial3)
FIND_PACKAGE(KDE4 REQUIRED)
INCLUDE_DIRECTORIES( ${KDE4_INCLUDES} )
SET(tutorial3_SRCS
main.cpp
mainwindow.cpp
)
KDE4_ADD_EXECUTABLE(tutorial3 ${tutorial3_SRCS})
TARGET_LINK_LIBRARIES( tutorial3 ${KDE4_KDEUI_LIBS})
install(TARGETS tutorial3 DESTINATION ${BIN_INSTALL_DIR})
install( FILES tutorial3ui.rc DESTINATION ${DATA_INSTALL_DIR}/tutorial3 )