Development/Tutorials/Using KActions
Development/Tutorials/Using_KActions
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Beginner Tutorial |
Previous | Tutorial 2 - KXmlGuiWindow, Basic XML knowledge |
What's Next | Tutorial 4 - Saving and loading |
Further Reading | None |
Abstract
This tutorial introduces the concept of actions. Actions are a unified way of supplying the user with ways to interact with your program.
For example, if we wanted to let the user of Tutorial 2 clear the text box by clicking a button in the toolbar, from an option in the File menu or through a keyboard shortcut, it could all be done with one KAction.
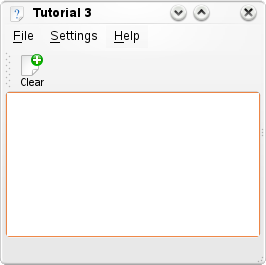
KAction
A KAction is an object which contains all the information about the icon and shortcuts that is associated with a certain action. The action is then connected to a slot which carries out the work of your action.
The Code
main.cpp
- include <KApplication>
- include <KAboutData>
- include <KCmdLineArgs>
- include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial3", "tutorial3",
ki18n("Tutorial 3"), "1.0",
ki18n("A simple text area using KAction etc."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
This time, very little has changed in main.cpp, only the KAboutData constructor has been updated to show that we are now on tutorial 3.
mainwindow.h
- ifndef MAINWINDOW_H
- define MAINWINDOW_H
- include <KXmlGuiWindow>
- include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
void setupActions();
};
- endif
Only a function void setupActions() has been added which will do all the work setting up the KActions.
mainwindow.cpp
- include "mainwindow.h"
- include <KApplication>
- include <KAction>
- include <KLocale>
- include <KActionCollection>
- include <KStandardAction>
MainWindow::MainWindow(QWidget *parent)
: KXmlGuiWindow(parent)
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KAction* clearAction = new KAction(this);
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("document-new"));
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
actionCollection()->addAction("clear", clearAction);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()),
actionCollection());
setupGUI();
}
Explanation
This builds upon the KXmlGuiWindow code from Tutorial 2. Most of the changes are to mainwindow.cpp, an important structural change being that the constructor for MainWindow now calls setupActions() instead of setupGUI(). setupActions() is where the new KAction code goes before finally calling setupGUI() itself.
Creating the KAction object
The KAction is built up in a number of steps. The first is including the KAction library and then creating the KAction:
- include <KAction>
...
KAction* clearAction = new KAction(this);
This creates a new KAction called clearAction.
Setting KAction Properties
Text
Now we have our KAction object, we can start setting its properties. The following code sets the text that will be displayed in the menu and under the KAction's icon in the toolbar.
clearAction->setText(i18n("Clear"));
Note that the text is passed through the i18n() function; this is necessary for the UI to be translatable (more information on this can be found in the i18n tutorial).
Icon
If the action is going to be displayed in a toolbar, it's nice to have an icon depicting the action. The following code sets the icon to the standard KDE document-new icon through the use of the setIcon() function:
clearAction->setIcon(KIcon("document-new"));
Keyboard Shortcut
Setting a keyboard shortcut to perform our action is equally simple:
clearAction->setShortcut(Qt::CTRL + Qt::Key_W);
This associates Ctrl+W with the KAction.
Adding to the Collection
In order for the action to be accessed by the XMLGUI framework (explained in depth later) it must be added to the application's action collection. The action collection is accessed via the actionCollection() function like this:
actionCollection()->addAction("clear", clearAction);
Here, the clearAction KAction is added to the collection and given a name of clear. This name (clear) is used by the XMLGUI framework to refer to the action, ergo, it should not be localized, since it is used internally only.
Connecting the action
Now that the action is fully set up, it needs to be connected to something useful. In this case (because we want to clear the text area), we connect our action to the clear() action belonging to a KTextEdit (which, unsurprisingly, clears the KTextEdit)
connect( clearAction, SIGNAL( triggered(bool) ),
textArea, SLOT( clear() ) );
This is the same as it would be done in Qt with a QAction.
KStandardAction
For actions which would likely appear in almost every KDE application such as 'quit', 'save', and 'load' there are pre-created convenience KActions, accessed through KStandardAction.
They are very simple to use. Once the library has been included (#include <KStandardAction>), simply supply it with what you want the function to do and which KActionCollection to add it to. For example:
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
This creates a KAction with the correct icon, text and shortcut and even adds it to the File menu.
At the moment, the new "Clear" action has been created but it hasn't been associated with any menus or toolbars. This is done with a KDE technology called XMLGUI, which does nice things like movable toolbars for you.
XMLGUI
The setupGUI() function in KXmlGuiWindow depends on the XMLGUI system to construct the GUI, which XMLGUI does by parsing an XML file description of the interface.
The rule for naming this XML file is appnameui.rc, where appname is the name you set in KAboutData (in this case, tutorial3). So in our example, the file is called tutorial3ui.rc, and is located in the build directory. Where the file will ultimately be placed is handled by CMake.
appnameui.rc File
Since the description of the UI is defined with XML, the layout must follow strict rules. This tutorial will not go into great depth on this topic, but for more information, see the detailed XMLGUI page (here is an older tutorial: [1]).
tutorial3ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE kpartgui SYSTEM "kpartgui.dtd">
<gui name="tutorial3" version="1">
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
<MenuBar>
<Menu name="file" >
<Action name="clear" />
</Menu>
</MenuBar>
</gui>
The <Toolbar> tag allows you to describe the toolbar, which is the bar across the top of the window normally with icons. Here it is given the unique name mainToolBar and its user visible name set to Main Toolbar using the <text> tag. The clear action is added to the toolbar using the <Action> tag, the name parameter in this tag being the string that was passed to the KActionCollection with addAction() in mainwindow.cpp.
Besides having the action in the toolbar, it can also be added to the menubar. Here the action is being added to the File menu of the MenuBar the same way it was added to the toolbar.
Change the 'version' attribute of the <gui> tag if you changed .rc file since the last install to force a system cache update.
Some notes on the interaction between code and the .rc file: Menus appear automatically and should have a <text/> child tag unless they refer to standard menus. Actions need to be created manually and inserted into the actionCollection() using the name in the .rc file. Actions can be hidden or disabled, whereas menus can't.
CMake
Finally, the tutorial3ui.rc needs to go somewhere where KDE can find it (can't just leave it in the source directory!). This means the project needs to be installed somewhere.
CMakeLists.txt
project(tutorial3)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(tutorial3_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial3 ${tutorial3_SRCS})
target_link_libraries(tutorial3 ${KDE4_KDEUI_LIBS})
install(TARGETS tutorial3 DESTINATION ${BIN_INSTALL_DIR})
install(FILES tutorial3ui.rc
DESTINATION ${DATA_INSTALL_DIR}/tutorial3)
This file is almost identical to the one for tutorial2, but with two extra lines at the end that describe where the files are to be installed. Firstly, the tutorial3 target is installed to the BIN_INSTALL_DIR then the tutorial3ui.rc file that describes the layout of the user interface is installed to the application's data directory.
Make, Install And Run
If you don't have write access to where your KDE4 installation directory, you can install it to a folder in your home directory.
To tell CMake where to install the program, set the DCMAKE_INSTALL_PREFIX switch. You probably just want to install it somewhere local for testing (it's probably a bit silly to go to the effort of installing these tutorials to your KDE directory), so the following might be appropriate:
mkdir build && cd build cmake .. -DCMAKE_INSTALL_PREFIX=$HOME make install $HOME/bin/tutorial3
which will create a KDE-like directory structure in your user's home directory directory and will install the executable to $HOME/bin/tutorial3.
Moving On
Now you can move on to saving and loading.