Development/Tutorials/Using KActions: Difference between revisions
m (→CMakeLists.txt) |
m (Added Language Navigation Bar) |
||
Line 1: | Line 1: | ||
{{Template:I18n/Language Navigation Bar|Development/Tutorials/Using_KActions}} | |||
{{TutorialBrowser| | {{TutorialBrowser| | ||
Revision as of 19:51, 4 August 2007
Development/Tutorials/Using_KActions
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Beginner Tutorial |
Previous | Tutorial 2 - KXmlGuiWindow, Basic XML knowledge |
What's Next | TODO (milliams) |
Further Reading | None |
Abstract
We're going to introduce the concept of actions. Actions are a unified way of supplying the user with ways to interact with your program.
Say, for example, we want to let the user clear the text box by clicking a button in the toolbar, from an option in the File menu or through a keyboard shortcut; we can provide all of those through one KAction.
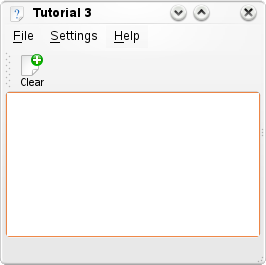
KAction
A KAction is an object which contains all the information about the icon and shortcuts you want associated to a certain action. You then connect the action to a slot which carries out the work of your action.
Creating Your Own
To create an action, you need to #include <KAction> in your .cpp file.
Creating the object
We're going to create an action which will clear the text area (see Tutorial 2). The KAction is built up in a number of steps. The first is creating the KAction
KAction* clearAction = new KAction(this);
This creates a KAction called clearAction.
Text
Now we have our KAction object, we can start setting its properties. First, we'll set the text that will be displayed in the menu and under its icon in the toolbar.
clearAction->setText(i18n("Clear"));
As you can see, the text must be passed through the i18n() function if you want your UI to be translatable.
Icon
If you're going to display the action in a toolbar, you're going to want to have an icon depicting the action. To set an icon we simply use the setIcon() function thus:
clearAction->setIcon(KIcon("filenew"));
Here we're setting the icon to the standard KDE filenew icon.
Shortcut
We can also set a shortcut that will perform our action. It's as simple as a
clearAction->setShortcut(Qt::CTRL+Qt::Key_W);
to set Ctrl+W to be associated to this action.
Adding to the Collection
In order for our action to be accessable by the XmlGui framework it must be added to the application's action collection. It is accessed via the actionCollection() function thus:
actionCollection()->addAction("clear", clearAction);
Here we add the clearAction KAction to the collection and give it a name of clear. This name is used by the XmlGui framework.
Connecting the action
Now our action is fully set up, we need to connect it to something useful. We're going to connect our action to the clear() action belonging to a KTextArea.
connect( clearAction, SIGNAL( triggered(bool) ),
textArea, SLOT( clear() ) );
This is the same as it would be done in Qt with a QAction.
KStandardAction
For actions which would likely appear in almost every KDE application such as 'quit', 'save', and 'load' there are pre-created convenience KActions, accessed through KStandardAction.
They are very simple to use. Once you've done #include <KStandardAction>, you simply need to supply it with what you want the function to do and which KActionCollection to add it to. For example,
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
Will Create a KAction with the correct icon, text and shortcut and will even add it to the File menu.
The Code
mainwindow.h
- ifndef MAINWINDOW_H
- define MAINWINDOW_H
- include <KXmlGuiWindow>
- include <KTextEdit>
class MainWindow : public KXmlGuiWindow
{
public:
MainWindow(QWidget *parent=0);
private:
KTextEdit* textArea;
void setupActions();
};
- endif
mainwindow.cpp
- include "mainwindow.h"
- include <KApplication>
- include <KAction>
- include <KLocale>
- include <KActionCollection>
- include <KStandardAction>
MainWindow::MainWindow(QWidget *parent)
: KXmlGuiWindow(parent)
{
textArea = new KTextEdit;
setCentralWidget(textArea);
setupActions();
}
void MainWindow::setupActions()
{
KAction* clearAction = new KAction(this);
clearAction->setText(i18n("Clear"));
clearAction->setIcon(KIcon("filenew"));
clearAction->setShortcut(Qt::CTRL+Qt::Key_W);
actionCollection()->addAction("clear", clearAction);
connect(clearAction, SIGNAL(triggered(bool)),
textArea, SLOT(clear()));
KStandardAction::quit(kapp, SLOT(quit()),
actionCollection());
setupGUI();
}
main.cpp
- include <KApplication>
- include <KAboutData>
- include <KCmdLineArgs>
- include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "tutorial3", "tutorial3",
ki18n("Tutorial 3"), "1.0",
ki18n("A simple text area using KAction etc."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
Now, at the moment, we've only created our new "Clear" action. It won't yet show up in the menus or in the toolbars. To tell the program where to put our actions (and to allow the end-user to move them around) we use a KDE technology called XmlGui.
XmlGui
When you call setupGUI() in your KXmlGuiWindow class, it calls the XmlGui system which reads an XML file description of your interface (which we will create in a minute) and creates the buttons and menus appropriately.
Now obviously XmlGui needs to know which file is your description file, i.e. it needs to know its name and location. The rule for the naming is the file should be called appnameui.rc (where appname is the name you set in KAboutData), so in our example, the file will be called tutorial3ui.rc. Where the file will be located is handled by CMake.
Writing your appnameui.rc File
Since the description of our UI is being defined with XML, the layout of the description must follow strict rules. We won't go through all the rules in this tutorial but for more information, see the _detailed_XmlGui_page_ (once we have a full explanation of XmlGui (or possibly liveui if that's done soon :)) on the wiki, I'll link it up).
tutorial3ui.rc
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE kpartgui SYSTEM "kpartgui.dtd">
<gui name="tutorial3" version="1">
<ToolBar name="mainToolBar" >
<text>Main Toolbar</text>
<Action name="clear" />
</ToolBar>
<MenuBar>
<Menu name="file" >
<text>&File</text>
<Action name="clear" />
</Menu>
</MenuBar>
</gui>
The <Toolbar> tag allows you to describe the toolbar. That is the bar across the top of the window with the icons. Here we give it a unique name mainToolBar, set it's user visible name Main Toolbar using the <text> tag and finally add our clear action to the toolbar using the <Action> tag. The name parameter in this tag relates to the string that was passed to the addAction() function in the C++ code.
As well as having our action in the toolbar, we can also add it to the menubar. Within the <MenuBar> tag, we say we want to add our action to the File menu and we add the action in the same way as for the toolbar.
Change 'version' attribute of the gui tag if you changed .rc file since last install to force system cache update
CMake
Now that we're using XmlGui, we need to put the tutorial3ui.rc somewhere where KDE can find it. This means we need to install our project somewhere.
CMakeLists.txt
project(tutorial3)
find_package(KDE4 REQUIRED)
include_directories( ${KDE4_INCLUDES} )
set(tutorial3_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(tutorial3 ${tutorial3_SRCS})
target_link_libraries(tutorial3 ${KDE4_KDEUI_LIBS})
install(TARGETS tutorial3 DESTINATION ${BIN_INSTALL_DIR})
install( FILES tutorial3ui.rc
DESTINATION ${DATA_INSTALL_DIR}/tutorial3 )
This file is almost identical to the one for tutorial2 but it has two extra lines at the end. These describe where the files are to be installed. Firstly, the tutorial3 target is installed to the BIN_INSTALL_DIR then the tutorial3ui.rc file that describes the layout of the user interface is installed to the application's data directory.
Make, Install And Run
If you don't have write access to where your KDE4 installation directory, you can install it to a folder in your home directory.
To tell CMake where to install the program, set the DCMAKE_INSTALL_PREFIX switch. So to install the program to the KDE directory, do
cmake . -DCMAKE_INSTALL_PREFIX=$KDEDIR make install tutorial3
Though, if you just want to install it somewhere local for testing (it's probably a bit silly to go to the effort of installing these tutorials to your KDE directory) you can do something like
cmake . -DCMAKE_INSTALL_PREFIX=/home/kde-devel/kdetmp
which will create a KDE-like directory structure under ~/kdetmp and will install the executable to /home/kde-devel/kdetmp/bin/tutorial3.
Moving On
TODO