Development/Tutorials/Qt4 Ruby Tutorial/Chapter 13/fi
Development/Tutorials/Qt4 Ruby Tutorial/Chapter 13
Languages: عربي | Asturianu | Català | Česky | Kaszëbsczi | Dansk | Deutsch | English | Esperanto | Español | Eesti | فارسی | Suomi | Français | Galego | Italiano | 日本語 | 한국어 | Norwegian | Polski | Português Brasileiro | Română | Русский | Svenska | Slovenčina | Slovenščina | српски | Türkçe | Tiếng Việt | Українська | 简体中文 | 繁體中文
Tutorial Series | Qt4 Ruby -oppikurssi |
Previous | Tutorial 12 - Hanging in the Air the Way Bricks Don't |
What's Next | Tutorial 14 - Facing the Wall |
Further Reading | n/a |
Peli loppui
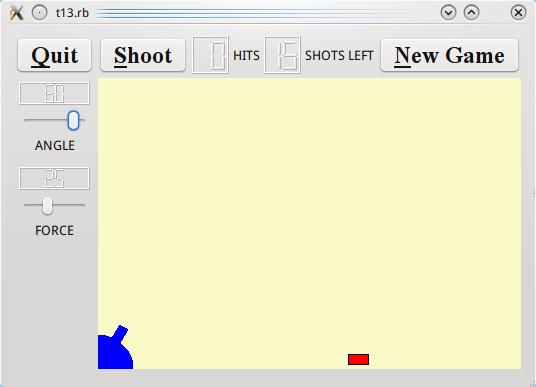
Files:
Yleiskuva
In this example we start to approach a real playable game with a score.
We give MyWidget a new name (GameBoard), add some slots, and move it to gamebrd.rb
The CannonField now has a game over state.
The layout problems in LCDRange are fixed.
Line by Line Walkthrough
@label.setSizePolicy(Qt::SizePolicy::Preferred, Qt::SizePolicy::Fixed)
We set the size policy of the Qt::Label to (Qt::SizePolicy::Preferred, Qt::SizePolicy::Fixed). The vertical component ensures that the label won't stretch or shrink vertically; it will stay at its optimal size (its QWidget::sizeHint()). This solves the layout problems observed in Chapter 12.
The CannonField now has a game over state and a few new functions.
signals 'canShoot(bool)'
This new signal indicates that the CannonField is in a state where the shoot() slot makes sense. We'll use it below to enable or disable the Shoot button.
@gameEnded = false
This variable contains the game state; true means that the game is over, and false means that a game is going on. Initially, the game is not over (luckily for the player :-).
def shoot()
if isShooting()
return
end
@timerCount = 0
@shootAngle = @currentAngle
@shootForce = @currentForce
@autoShootTimer.start(5)
emit canShoot(false)
end
We added a new isShooting() function, so shoot() uses it instead of testing directly. Also, shoot tells the world that the CannonField cannot shoot now.
def setGameOver()
if @gameEnded
return
end
if isShooting()
@autoShootTimer.stop()
end
@gameEnded = true
update()
end
This slot ends the game. It must be called from outside CannonField, because this widget does not know when to end the game. This is an important design principle in component programming. We choose to make the component as flexible as possible to make it usable with different rules (for example, a multi-player version of this in which the first player to hit ten times wins could use the CannonField unchanged).
If the game has already been ended we return immediately. If a game is going on we stop the shot, set the game over flag, and repaint the entire widget.
def restartGame()
if isShooting()
@autoShootTimer.stop()
end
@gameEnded = false
update()
emit canShoot(true)
end
This slot starts a new game. If a shot is in the air, we stop shooting. We then reset the gameEnded variable and repaint the widget.
moveShot() too emits the new canShoot(true) signal at the same time as either hit() or miss().
Modifications in CannonField::paintEvent():
def paintEvent(event)
painter = Qt::Painter.new(self)
if @gameEnded
painter.setPen(Qt::black)
painter.setFont(Qt::Font.new( "Courier", 48, Qt::Font::Bold))
painter.drawText(rect(), Qt::AlignCenter, tr("Game Over"))
end
The paint event has been enhanced to display the text "Game Over" if the game is over, i.e., gameEnded is true. We don't bother to check the update rectangle here because speed is not critical when the game is over.
To draw the text we first set a black pen; the pen color is used when drawing text. Next we choose a 48 point bold font from the Courier family. Finally we draw the text centered in the widget's rectangle. Unfortunately, on some systems (especially X servers with Unicode fonts) it can take a while to load such a large font. Because Qt caches fonts, you will notice this only the first time the font is used.
paintCannon(painter)
if isShooting()
paintShot(painter)
end
unless @gameEnded
paintTarget(painter)
end
painter.end()
end
We draw the shot only when shooting and the target only when playing (that is, when the game is not ended).
This file is new. It contains the GameBoard class, which was last seen as MyWidget.
slots 'fire()', 'hit()', 'missed()', 'newGame()'
We have now added four slots.
We have also made some changes in the GameBoard constructor.
@cannonField = CannonField.new()
@cannonField is now a member variable, so we carefully change the constructor to use it.
connect(@cannonField, SIGNAL('hit()'),
self, SLOT('hit()'))
connect(@cannonField, SIGNAL('missed()'),
self, SLOT('missed()'))
This time we want to do something when the shot has hit or missed the target. Thus we connect the hit() and missed() signals of the CannonField to two protected slots with the same names in this class.
connect(shoot, SIGNAL('clicked()'), self, SLOT('fire()') )
Previously we connected the Shoot button's clicked() signal directly to the CannonField's shoot() slot. This time we want to keep track of the number of shots fired, so we connect it to a slot in this class instead.
Notice how easy it is to change the behavior of a program when you are working with self-contained components.
connect(@cannonField, SIGNAL('canShoot(bool)'),
shoot, SLOT('setEnabled(bool)'))
We also use the CannonField's canShoot() signal to enable or disable the Shoot button appropriately.
restart = Qt::PushButton.new(tr('&New Game'))
restart.setFont(Qt::Font.new('Times', 18, Qt::Font::Bold))
connect(restart, SIGNAL('clicked()'), self, SLOT('newGame()'))
We create, set up, and connect the New Game button as we have done with the other buttons. Clicking this button will activate the newGame() slot in this widget.
@hits = Qt::LCDNumber.new(2)
@shotsLeft = Qt::LCDNumber.new(2)
hitsLabel = Qt::Label.new(tr('HITS'))
shotsLeftLabel = Qt::Label.new(tr('SHOTS LEFT'))
We create four new widgets, to display the number of hits and shots left.
topLayout = Qt::HBoxLayout.new()
topLayout.addWidget(shoot)
topLayout.addWidget(@hits)
topLayout.addWidget(hitsLabel)
topLayout.addWidget(@shotsLeft)
topLayout.addWidget(shotsLeftLabel)
topLayout.addStretch(1)
topLayout.addWidget(restart)
The top-right cell of the Qt::GridLayout is starting to get crowded. We put a stretch just to the left of the New Game button to ensure that this button will always appear on the right side of the window.
newGame()
We're all done constructing the GameBoard, so we start it all using newGame(). Although newGame() is a slot, it can also be used as an ordinary function.
def fire()
if @cannonField.gameOver() || @cannonField.isShooting()
return
end
@shotsLeft.display(@shotsLeft.intValue() - 1)
@cannonField.shoot()
end
This function fires a shot. If the game is over or if there is a shot in the air, we return immediately. We decrement the number of shots left and tell the cannon to shoot.
def hit()
@hits.display(@hits.intValue() + 1)
if @shotsLeft.intValue() == 0
@cannonField.setGameOver()
else
@cannonField.newTarget()
end
end
This slot is activated when a shot has hit the target. We increment the number of hits. If there are no shots left, the game is over. Otherwise, we make the CannonField generate a new target.
def missed()
if @shotsLeft.intValue() == 0
@cannonField.setGameOver()
end
end
This slot is activated when a shot has missed the target. If there are no shots left, the game is over.
def newGame()
@shotsLeft.display(15)
@hits.display(0)
@cannonField.restartGame()
@cannonField.newTarget()
end
This slot is activated when the user clicks the New Game button. It is also called from the constructor. First it sets the number of shots to 15. Note that this is the only place in the program where we set the number of shots. Change it to whatever you like to change the game rules. Next we reset the number of hits, restart the game, and generate a new target.
This file has just been on a diet. MyWidget is gone, and the only thing left is the main() function, unchanged except for the name change.
Running the Application
The cannon can shoot at a target; a new target is automatically created when one has been hit.
Hits and shots left are displayed and the program keeps track of them. The game can end, and there's a button to start a new game.
Exercises
Add a random wind factor and show it to the user.
Make some splatter effects when the shot hits the target.
Implement multiple targets.