Development/Tutorials/Physical Simulation
Parts to be reviewed:
Port to KF5 and KDevelop5Introduction
Often in engineering you want to animate things, and KDE has a great method for doing this. I found KDevelop particular helpful due to the auto-completion. However, this tutorial also gives you the vi/emacs/randomEditor version.
Since the code will become relatively long for a tutorial, the tutorial is split into sections. At the end of each section, the program will produce something.
In this tutorial you will learn how to:
- get two or more widgets in one window
- plot data in an xy-plot
- draw something on a widget
- animate something engineering / physics based. What is easier than a bouncing ball
- use KDevelop if you choose to so (the inside help system and link to the KDE and QT help are great)
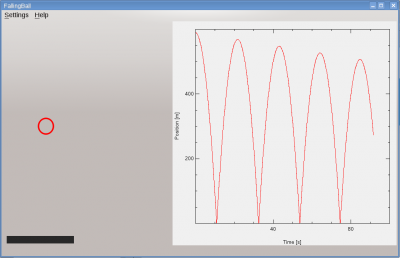
Setup
- In KDevelop:
Open CMakeLists.txt by double clicking on it.
- In a Text Editor
- Make directory and create a CMakeLists.txt file, which cmake requires to find dependencies and compile the program.
project (FallingBall)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(FallingBall_SRCS
main.cpp
FallingBall.cpp
)
kde4_add_executable(FallingBall ${FallingBall_SRCS})
target_link_libraries(FallingBall ${KDE4_KDEUI_LIBS})
The first line gives a name to the project, and the second ensures that the klibs are installed; while the third sets the header files appropriately. The "set" line is the most important. We have to put in all the ".cpp" files that we use in here. For now, just add the main.cpp and the FallingBall.cpp. Others will join later. Finally, define the executable and link all files.
Basic structure
Now lets start with main.cpp:
This is just the starter point of the application, which starts the main window.
Include conventional headers, which allow to create a KDE application, a nice AboutBox and handle command line arguments.
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
//Include the header of the new main window.
#include "FallingBall.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "FallingBall", 0,
ki18n("FallingBall"), "1.0",
ki18n("This is some random text"),
KAboutData::License_GPL,
ki18n("Copyright (c) 2012 Your name") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
FallingBall* mainWindow = new FallingBall();
mainWindow->show();
return app.exec();
}
The header files should be self-explanatory. Of special interest is the second part. We create a new application by the name of "app". Then we create a new main window from the type FallingBall. The central things will happen in this class, hence mainWindow. After creation, we show the mainWindow and execute the app, which waits for user interactions, does the repainting, ...
Now lets come to FallingBall.h. The header for the main program.
#ifndef FallingBall_H
#define FallingBall_H
#include <KXmlGuiWindow>
#include <QTimer>
#include <KPlotWidget>
class FallingBall : public KXmlGuiWindow
{
public:
FallingBall(QWidget *parent=0);
private:
QWidget *master;
KPlotWidget *xyPlot;
};
#endif // FallingBall_H
This file will be expanded later on. Lets keep it simple for now, such that we can test the current version and are not bugged down in many interrelated bugs.
The KXmlGuiWindow allows us fancy application. The Qtimer is required for the motion of the ball and the KPlotWidget shows us the graph of the data. This main window is a child of the KXmlGuiWindow. and has a constructor with a default value of 0, which implies it is the main window. We also add one QWidget, which will allow us to position multiple things on the main window. Also we add already the KPlotWidget, since this widget is debugged and working nicely.
Now lets come to the main window: FallingBall.cpp:
#include "FallingBall.h"
#include <QHBoxLayout>
FallingBall::FallingBall(QWidget *parent) : KXmlGuiWindow(parent)
{
master = new QWidget();
xyPlot = new KPlotWidget();
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(xyPlot);
master->setLayout(layout);
setCentralWidget(master);
setupGUI();
QTimer *timer = new QTimer(this);
timer->start(100);
}
We include the appropriate header and the QHBoxLayout, which allows us to place multiple widgets in the main window. We create a new master QWidget, which will hold the picture of the falling ball and the graph. Then, we create an instance of the KPlotWidget. We define a new layout to place the things on the master. Here we choose horizontal layout but vertical and grid layout exist. Which can be further divided. Therefore, almost endless possibilities.
We add the xyPlot widget to the layout for now. With only one well tested widget, we can test our code.
Next we assign the layout to the master widget. We can only assign layouts to widgets, even empty once. This main window is not a QWidget, hence we cannot assign a layout to it.
After the master is setup, we can tell the compiler that this master should be the central widget and setup the GUI, which does some administrative stuff.
Finally, we define a new timer which will support the animation and we set the refresh rate to 100 milliseconds.
Now we should be able to start the application.
Now lets test this minimal program:
- In KDevelop
- Compile with F8
- setup the execution by Run->Configure Launches. Click on the Name "Falling Ball" and add "+" a launch to it.
- configure the launch by clicking on the Project Target: FallingBall/FallingBall
- and OK. From now on you can just hit ... (If you have multiple open projects, you must switch between different launches by choosing the project under "Run-Current Launch Configuration)
- F9
- In Editor
cmake . && make && ./FallingBall
Adding physics
Now lets create the ball logic, which calculates the position,... . No drawing is done here, that will be done in the ball widget later. Always remember, use small (but meaningful) building blocks. That way you can test them and find problems fast.
- BallLogic.h
#ifndef _BALL_LOGIC_H_
#define _BALL_LOGIC_H_
#include <QWidget>
class BallLogic : public QWidget {
Q_OBJECT
public:
BallLogic(double dTime=0.1);
double getPosition();
double getTime();
private:
double position;
double velocity;
double acceleration;
double time,dTime;
public slots:
void nextTimeStep();
};
#endif
This might seem strange: if we just want to put the logic in here, no drawing, why would we need a QWidget? Answer, the logic includes the next time step, which is a so called slot. We discuss the slots later. Only this much here. Since we want to have a "slot" we require to inherit from QWidget, which has slots. This slot must be public, i.e. we want to access it from outside this class.
The Q_OBJECT is a macro, which will setup some default things.
The constructor requires a time step in seconds while the getPosition returns the current position. The private variables are self explanatory.
Now lets go into the ball logic code BallLogic.cpp:
#include "BallLogic.h"
BallLogic::BallLogic(double dTime) {
this->dTime = dTime;
acceleration = -9.81;
velocity = 0;
position = 590;
time=0;
}
double BallLogic::getPosition() {
return position;
}
double BallLogic::getTime() {
return time;
}
void BallLogic::nextTimeStep() {
time += dTime;
if(position<=0 && velocity<0) velocity=-velocity;
velocity += acceleration*dTime;
position += velocity*dTime+0.5*acceleration*dTime*dTime;
}
The constructor sets the time step of this class and defines the acceleration constant, together with some starting conditions: we start at pixel, with zero velocity at time zero. The getPosition method returns the current position and the next time step does the calculation. If the current position and velocity are negative, the ball is hitting the floor and has to bounce back. (Omitting the velocity condition might result in a strange behavior.) Bouncing back implies a reversal of the velocity. The new velocity and position are calculated according to Newtonian mechanics. Check the corresponding Wikipedia if you like.
Now lets test it again. Frequent testing is the path to success. First add the new BallLogic.cpp to the CMakeLists.txt! Then, if you use KDevelop "F8" and "F9". Else use make and execute the application.
Adding drawing functions
After the logic is done, now it is time to do the drawing. First the header file.
#ifndef _BALL_GRAPH_H_
#define _BALL_GRAPH_H_
#include <QWidget>
#include <KPlotWidget>
#include <KPlotObject>
#include <KPlotAxis>
#include <QtGui>
#include "BallLogic.h"
class BallGraph : public QWidget {
Q_OBJECT
public:
BallGraph(BallLogic *logic, KPlotWidget *xyPlot, QWidget *parent = 0);
QSize minimumSizeHint() const;
QSize sizeHint() const;
protected:
BallLogic *logic;
KPlotWidget *xyPlot;
KPlotObject *xyData;
void paintEvent(QPaintEvent *event);
double radius;
public slots:
void nextAnimationFrame();
};
#endif
Our ball drawing widget will inherit from QWidget, which is an feasible background. As such it requires again the Q_OBJECT macro, see before.
Now we come to a small detail. Our drawing widget needs to have a pointer to the xy-plot widget such that it can post data there. Moreover, the drawing widget requires a pointer to the ball logic, such that it can inquire about the position of the ball at the current time. All three widgets need to talk to each other, therefore a pointer is required which allows for communication, xyPlot.
To allow for usage of the xy-plot (KPlotWidget) three header files are included. The second defines the data structure, which can be plotted. This data structure is again a pointer called xyData.
The radius defines the radius of the ball, and paintEvent and nextAnimationFrame allow for drawing of the next time frame. The paintEvent, which does the drawing, is called whenever the update() method is called. The nextAnimationFrame only calls all the update methods. (The update method is inherited from QWidget).
minimumSizeHint and sizeHint specify the minimum and suggested size of this widget. Remember, this widget is only the drawing widget on the right hand side. They could be different, but lazy people as me set them equal.
Now, on to the BallGraph.cpp
#include "BallGraph.h"
BallGraph::BallGraph(BallLogic* logic, KPlotWidget* xyPlot, QWidget* parent): QWidget(parent) {
this->logic = logic;
radius = 20;
setBackgroundRole(QPalette::Base); //Background color: this nice gradient of gray
setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Expanding); //allow for expanding of the widget, aka use the minimumSizeHint and sizeHint
// For xy plot using KplotWidget
this->xyPlot = xyPlot; //set pointer
xyPlot->setMinimumSize( 600, 600 ); //set minimum size in pixel
xyData = new KPlotObject( Qt::red, KPlotObject::Lines, 2 ); //the data lines are drawn in red with thickness 2
xyPlot->setLimits(0, 100, 0, 600); //the data limits are 0 to 100 in the x-direction and 0 to 600 in the y-direction (initially we start at point 590)
xyPlot->addPlotObject(xyData); //assign the data pointer to the graph. From now on, if the data pointer contains data, it is plotted in the graph
xyPlot->setBackgroundColor( QColor(240, 240, 240) ); //background: light shade of gray
xyPlot->setForegroundColor( Qt::black ); //foreground: black
xyPlot->axis( KPlotWidget::BottomAxis )->setLabel("Time [s]"); //set x-axis labeling
xyPlot->axis( KPlotWidget::LeftAxis )->setLabel("Position [m]"); //set y-axis labeling
}
QSize BallGraph::minimumSizeHint() const {
return QSize(200, 600);
}
QSize BallGraph::sizeHint() const {
return QSize(200, 600);
}
void BallGraph::nextAnimationFrame() {
double time=logic->getTime();
double position=logic->getPosition();
xyData->addPoint(time, position);
xyPlot->update();
update();
}
void BallGraph::paintEvent(QPaintEvent* event) {
double position=logic->getPosition();
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing, true);
//draw ball
painter.setPen(QPen(Qt::red, 4));
painter.drawEllipse(95, 575-position, 2*radius, -2*radius);
//draw floor
painter.fillRect( 10, 595, 180, -20 , QColor(40, 40, 40) );
}
The constructor connects the pointers of the ball logic and the KplotWidget to the current class and sets the radius. The remainder of the constructor is explained with the comments.
The minimumSizeHint and sizeHint were discussed before.
During each animation time step, the current physical time and ball position is queried from the ball logic class. This data is added to the data stream, which will be plotted in the xy-Plot using KPlotWidget.
The pointer is used to call the update method. Finally, the update, aka drawing, method of this class is called.
During the drawing in paint event, first the current position of the ball in inquired using the pointer. Then we use a painter, to draw on this ball drawing widget. Clearly, everybody wants to use Antialiasing.
Then we draw the ball using a red color and a 4 pixel wide pen. We draw an ellipse with the given boundary box. First the bottom left coordinate of the bounding rectangle is given. Be aware, the computer screens have the zero position at the top left corner, not as most people would expect at the bottom left corner. Therefore, we need to calculate the y-positions by some negative signs.
The total window height is 600, drawing the floor requires 25, therefore, 575-position gives the bottom coordinate. The ball has twice the radius diameter. Since the y-coordinates count from the top side, we have to use a minus again.
Finally we draw the floor in dark gray with a filled rectangle of given dimensions: 180 wide and 20 pixel high while the bottom left corner has the given coordinates.
Assembling
Now we need to wire everything together in FallingBall.cpp and FallingBall.h First lets do the header file, which should look like this now:
#ifndef FallingBall_H
#define FallingBall_H
#include <KXmlGuiWindow>
#include <QTimer>
#include <KPlotWidget>
#include "BallGraph.h"
#include "BallLogic.h"
class FallingBall : public KXmlGuiWindow
{
public:
FallingBall(QWidget *parent=0);
private:
QWidget *master;
BallLogic *logic;
KPlotWidget *xyPlot;
BallGraph *ballGraph;
};
#endif // FallingBall_H
We include the header files for the logic and the graph and add two pointers for the two widgets in the private section.
Now to the wiring in the FallingBall.cpp:
#include "FallingBall.h"
#include <QHBoxLayout>
FallingBall::FallingBall(QWidget *parent) : KXmlGuiWindow(parent)
{
master = new QWidget();
xyPlot = new KPlotWidget();
logic = new BallLogic();
ballGraph = new BallGraph(logic, xyPlot);
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(ballGraph);
layout->addWidget(xyPlot);
master->setLayout(layout);
setCentralWidget(master);
setupGUI();
QTimer *timer = new QTimer(this);
connect(timer, SIGNAL(timeout()),
logic, SLOT( nextTimeStep() ) );
connect(timer, SIGNAL(timeout()),
ballGraph, SLOT(nextAnimationFrame()));
timer->start(100);
}
Mostly, it is the same as before, now we only define the pointers for the xy-plot and the ball-logic and give those pointers to the constructor of the ball-drawing widget. We add the drawing widget to the layout. The widgets are added from the left to the right: the first goes left, the second goes to the right of that and so on.
Finally, we start the animation with some connect action. First we connect the signal / event of the timer reaching the timeout (after 100ms), to the logic -> next time step. I envision this as a signal comes from a source (timer) and reaches a destination (ball logic) at a given slot. This event triggers the nextTimeStep method defined in the BallLogic class.
Similarly whenever the 100ms are passed, the ballGraph gets a signal to add a new data point to the xy-Data and draw it using the update method of KPlotWidget and then update itself, draw the new ball and floor.
With the updated CMakeLists.txt: we are done.
set(FallingBall_SRCS
main.cpp
FallingBall.cpp
BallLogic.cpp
BallGraph.cpp
)
If you made no mistake, you should get a application that looks similar to the one on the top.
Extensions
