Development/Tutorials/Games/Highscores
Tutorial Series | KDE Games |
Previous | Introduction to KDE4 programming, KStandardGameAction Tutorial |
What's Next | |
Further Reading | KScoreDialog |
Abstract
This tutorial will explain how to use the high score system built into kdegames in your application.
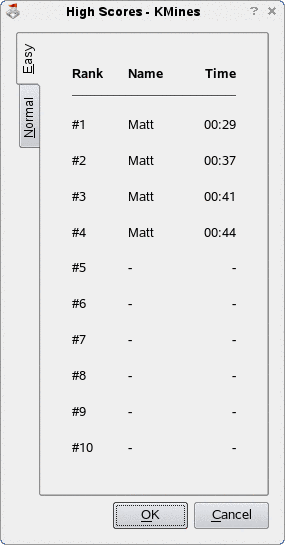
KScoreDialog
The high score system is provided through the KScoreDialog class. It is intended to be a lightweight solution and offers the following abilities:
- Grouping of scores (for different difficulty levels etc.)
- Standard fields: Name, Level, Date, Time, Score
- Custom fields (e.g. number of moves)
Displaying Scores
To display the high score table, it's as simple as two lines of code.
- include <KScoreDialog>
void showHighscores()
{
KScoreDialog ksdialog(KScoreDialog::Name, this);
ksdialog.exec();
}
This will display the highscore table showing, by default, the 'Name' and 'Score' columns.
In order to comply with the kdegames standards, you should use a KStandardGameAction to launch this function. This will set up the icons and toolbars correctly. See the KStandardGameAction tutorial for more information on this.
- include <KStandardGameAction>
void setupActions()
{
KStandardGameAction::highscores(this,
SLOT(showHighscores()),
actionCollection());
setupGUI();
}
Adding Scores
Simplest way to add a score
The simplest way to add a new score to the table is as follows:
- include <KScoreDialog>
void newHighscore(int playersScore)
{
KScoreDialog ksdialog(KScoreDialog::Name, this);
ksdialog.addScore(playersScore);
ksdialog.exec();
}
This will add the score playersScore to the high score table and then launch the dialog. If it is the first score to be entered on the table, a blank line-edit will be provided for the player to enter their name, otherwise the line-edit will still be provided but the player's name will be automatically filled in.
However, you will notice that the dialog is shown every time, even if the player didn't manage to get onto the table. If you only want to show the table if the player achieved a high score, place an if() command around the KScoreDialog::addScore() call:
if(ksdialog.addScore(playersScore))
ksdialog.exec();
Passing name by code
If you want to suggest a name programatically you can do so by using the KScoreDialog::FieldInfo type. It's easy to use, for example:
- include <KScoreDialog>
void newHighscore(int score, const QString& name)
{
KScoreDialog ksdialog(KScoreDialog::Name, this);
KScoreDialog::FieldInfo scoreInfo;
scoreInfo[KScoreDialog::Name] = name;
scoreInfo[KScoreDialog::Score].setNum(score);
ksdialog.addScore(scoreInfo);
}
And in this same way you can display other default fields by passing them to the KScoreDialog constructor and adding the information to your KScoreDialog::FieldInfo. For example, to display the level the player got to:
- include <KScoreDialog>
void newHighscore(int score, const QString& name, int level)
{
KScoreDialog ksdialog(KScoreDialog::Name |
KScoreDialog::Level, this);
KScoreDialog::FieldInfo scoreInfo;
scoreInfo[KScoreDialog::Name] = name;
scoreInfo[KScoreDialog::Score].setNum(score);
scoreInfo[KScoreDialog::Level].setNum(level);
ksdialog.addScore(scoreInfo);
}
Custom fields
Since there are only a set number of standatd fields it is possible to add you own custom fields. For example you may want to put the number of moves the player made on the table. This is done through the KScoreDialog::addField() function.
- include <KScoreDialog>
void newHighscore(int score, const QString& name, int numMoves)
{
KScoreDialog ksdialog(KScoreDialog::Name, this);
KScoreDialog::FieldInfo scoreInfo;
scoreInfo[KScoreDialog::Name] = "Matt";
scoreInfo[KScoreDialog::Score].setNum(playersScore);
ksdialog.addField(KScoreDialog::Custom1,
i18n("Num of Moves"), "moves");
scoreInfo[KScoreDialog::Custom1].setNum(numMoves);
ksdialog.addScore(scoreInfo);
}
The first argument to KScoreDialog::addField() must be from KScoreDialog::Custom1 to KScoreDialog::Custom5, the second is the text that will appear on the high score table at the head of the column (and must therefore be i18n'd) and the last argument is a unique identifier for the field. After that, it is added to your KScoreDialog::FieldInfo in the same way as before.
Grouping Scores
Your game may have different levels of difficulty and so you don't want to put the scores for the 'Hard' level on the same table as the 'Easy' table. Grouping scores is easy to achieve using the KScoreDialog::setConfigGroup() function:
- include <KScoreDialog>
void newHighscore(int playersScore, const QString& difficulty)
{
KScoreDialog ksdialog(KScoreDialog::Name, this);
ksdialog.setConfigGroup(difficulty);
ksdialog.addScore(playersScore);
ksdialog.exec();
}
Each group will show up as a tab on the high score table dialog. If you don't specify a group, the score will be added to the generic 'High Score' tab.
Advanced Features
Hiding Fields
In some special cases you won't want to display a score as such but rather just the time the player took to complete the level.
While you're not going to display the 'Score' field in your table you must always submit a value to 'Score' field since it is used for sorting the scores. In fact, the 'Score' field is always present in a KScoreDialog object even if you didn't pass it to the constructor. However, ity is possible to hide the 'Score' field (and in fact any field) from display using the KScoreDialog::hideField() function. It takes a Field flag from the same set that the constructor takes.
- include <KScoreDialog>
// timeString is a nicely formatted string
// e.g. "1:24" used for display
void newHighscore(const QString& timeString, int seconds)
{
KScoreDialog ksdialog(KScoreDialog::Name |
KScoreDialog::Time, this);
KScoreDialog::FieldInfo scoreInfo;
scoreInfo[KScoreDialog::Time] = timeString;
scoreInfo[KScoreDialog::Score].setNum(seconds);
ksdialog.hideField(KScoreDialog::Score);
ksdialog.addScore(scoreInfo, KScoreDialog::LessIsMore)
ksdialog.exec();
}