Archive:Development/Tutorials/First program (zh CN): Difference between revisions
(→摘要) |
(→代码) |
||
Line 28: | Line 28: | ||
== 代码 == | == 代码 == | ||
我们需要的全部代码都在一个文件<tt>main.cpp</tt>中。使用下列代码创建该文件: | |||
<code cppqt> | <code cppqt> | ||
#include <QString> | #include <QString> | ||
Line 39: | Line 39: | ||
int main (int argc, char *argv[]) | int main (int argc, char *argv[]) | ||
{ | { | ||
KAboutData aboutData("tutorial1", // | KAboutData aboutData("tutorial1", // 内部使用的程序名 | ||
0, // | 0, // 消息编目名。如果为null,则使用程序名 | ||
ki18n("Tutorial 1"), // | ki18n("Tutorial 1"), // 显示用的程序名称字符串 | ||
"1.0", // | "1.0", // 程序版本号字符串 | ||
ki18n("KMessageBox popup"), // | ki18n("KMessageBox popup"), // 对程序实现功能的简要描述 | ||
KAboutData::License_GPL, // | KAboutData::License_GPL, // 授权标识符 | ||
ki18n("(c) 2007"), // | ki18n("(c) 2007"), // 版权声明 | ||
ki18n("Some text..."), // | ki18n("Some text..."), // 任意格式的文本,可以包含任意类型的信息 | ||
"http://tutorial.com", // | "http://tutorial.com", // 程序主页字符串 | ||
"[email protected]"); // | "[email protected]"); // 报告bug用的邮件地址字符串 | ||
KCmdLineArgs::init( argc, argv, &aboutData ); | KCmdLineArgs::init( argc, argv, &aboutData ); | ||
Line 58: | Line 58: | ||
} | } | ||
</code> | </code> | ||
我们在此程序中遇到的第一个KDE类是{{class|KAboutData}}。这个类被用来保存关于程序的各种信息,如功能的简单描述、作者和版权信息等。几乎所有的KDE应用程序都会使用该类。 | |||
Then we come to {{class|KCmdLineArgs}}. This is the class one would use to specify command line switches to, for example, open the program with a specific file. However, in this tutorial, we simply initialise it with the {{class|KAboutData}} object we created so we can use the <tt>--version</tt> or <tt>--author</tt> switches. | Then we come to {{class|KCmdLineArgs}}. This is the class one would use to specify command line switches to, for example, open the program with a specific file. However, in this tutorial, we simply initialise it with the {{class|KAboutData}} object we created so we can use the <tt>--version</tt> or <tt>--author</tt> switches. |
Revision as of 12:36, 24 September 2007
Template:I18n/Language Navigation Bar (zh CN)
Tutorial Series | 初学者教程 |
Previous | C++, Qt, KDE4 开发环境 |
What's Next | 教程2 - 创建主窗口 |
Further Reading | CMake |
摘要
你的第一个程序将会用一句"Hello World"来向这个世界问好。为了实现它,我们将使用KMessageBox,并定制其中的一个按钮。
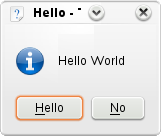
阅读此教程以正确设置KDevelop。你可以通过先用KDevelop试验打开一个已存在的KDE4应用程序来检验设置是否正确。
不过,你仍然需要手动编辑CMake文件。
代码
我们需要的全部代码都在一个文件main.cpp中。使用下列代码创建该文件:
- include <QString>
- include <KApplication>
- include <KAboutData>
- include <KMessageBox>
- include <KCmdLineArgs>
- include <KLocalizedString>
int main (int argc, char *argv[])
{
KAboutData aboutData("tutorial1", // 内部使用的程序名
0, // 消息编目名。如果为null,则使用程序名
ki18n("Tutorial 1"), // 显示用的程序名称字符串
"1.0", // 程序版本号字符串
ki18n("KMessageBox popup"), // 对程序实现功能的简要描述
KAboutData::License_GPL, // 授权标识符
ki18n("(c) 2007"), // 版权声明
ki18n("Some text..."), // 任意格式的文本,可以包含任意类型的信息
"http://tutorial.com", // 程序主页字符串
"[email protected]"); // 报告bug用的邮件地址字符串
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
KGuiItem guiItem( QString( "Hello" ), QString(),
QString( "this is a tooltip" ),
QString( "this is a whatsthis" ) );
KMessageBox::questionYesNo( 0, "Hello World", "Hello", guiItem );
}
我们在此程序中遇到的第一个KDE类是KAboutData。这个类被用来保存关于程序的各种信息,如功能的简单描述、作者和版权信息等。几乎所有的KDE应用程序都会使用该类。
Then we come to KCmdLineArgs. This is the class one would use to specify command line switches to, for example, open the program with a specific file. However, in this tutorial, we simply initialise it with the KAboutData object we created so we can use the --version or --author switches.
On line 13 we create a KApplication object. This needs to be done exactly once in each program since it is needed for things such as i18n.
Now we've done all the necessary KDE setup, we can move on to doing interesting things with our application. We're going to create a popup box but we're going to customise one of the buttons. To do this customisation, we need to use a KGuiItem object. The first argument in the KGuiItem constructor is the text that will appear on the item (in our case, a button). Then we have an option of setting an icon for the button but we don't want one so we just give it QString(). Finally we set the tooltip (what appears when you hover over an item) and finally the "What's This?" (accessed through right-clicking or Shift-F1) text.
Now we have our item, we can create our popup. we call the KMessageBox::questionYesNo() function which, by default, creates a message box with a "Yes" and a "No" button. The second argument is the text that will appear in the middle of the popup box. The third is the caption the window will have and finally we set the KGuiItem for (what would normally be) the "Yes" button to the KGuiItem guiItem we created.
We're all done as far as the code is concerned. Now to build it and try it out.
构建
If you set up your environment as described in Getting_Started/Build/Unstable_Version, you can compile this code with
g++ main.cpp -o tutorial1 \ -I$QTDIR/include/Qt \ -I$QTDIR/include/QtCore \ -I$QTDIR/include \ -I$KDEDIR/include/KDE \ -I$KDEDIR/include \ -L$KDEDIR/lib \ -L$QTDIR/lib -lQtCore -lQtGui -lkdeui -lkdecore
and then run it with
dbus-launch ./tutorial1
使用CMake
If that worked, you may want to use CMake, just like the rest of KDE. This will automatically locate the libraries and headers for KDE, Qt etc. and will allow you to easily build your applications on other computers.
CMakeLists.txt
Create a file named CMakeLists.txt in the same directory as main.cpp with this content:
project (tutorial1)
find_package(KDE4 REQUIRED)
include_directories( ${KDE4_INCLUDES} )
set(tutorial1_SRCS main.cpp)
kde4_add_executable(tutorial1 ${tutorial1_SRCS})
target_link_libraries(tutorial1 ${KDE4_KDEUI_LIBS})
The find_package() function locates the package that you ask it for (in this case KDE4) and sets some variables describing the location of the package's headers and libraries. In this case we will use the KDE4_INCLUDES variable which contains the path to the KDE4 header files.
In order to allow the compiler to find these files, we pass that variable to the include_directories() function which adds the KDE4 headers to the header search path.
Next we create a variable called tutorial1_SRCS using the set() function. In this case we simply set it to the name of our only source file.
Then we use kde4_add_executable() to create an executable called tutorial1 from the source files listed in our tutorial1_SRCS variable. Finally we link our executable to the KDE4 kdeui library using target_link_libraries() and the KDE4_KDEUI_LIBS variable which was set by the find_package() function.
Make与运行
Again, if you set up your environment as described in Getting Started/Build/KDE4, you can compile this code with:
cmakekde
And launch it as:
./tutorial1.shell
继续前进
Now you can move on to 创建主窗口.