Development/Tutorials/Plasma4/GettingStarted: Difference between revisions
Bgrolleman (talk | contribs) (Initital Setup of Plasmoid Tutorial) |
Bgrolleman (talk | contribs) m (→Abstract) |
||
Line 12: | Line 12: | ||
}} | }} | ||
==Abstract== | ==Abstract== | ||
Where going to start creating a simple plasmoid in this tutorial, to keep things simple we will only create a static plasmoid, it will contain the following items. | |||
* SVG Background | |||
* Icon | |||
* Some nice text | |||
[[image:creatingyourfirstplasmoid1.png|frame|center]] | [[image:creatingyourfirstplasmoid1.png|frame|center]] | ||
Revision as of 06:03, 6 July 2007
Tutorial Series | Plasma Tutorial |
Previous | C++, Qt, KDE4 development environment |
What's Next | |
Further Reading | CMake |
Abstract
Where going to start creating a simple plasmoid in this tutorial, to keep things simple we will only create a static plasmoid, it will contain the following items.
- SVG Background
- Icon
- Some nice text
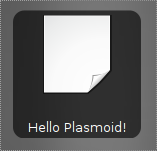
The Code
Plasmoids require a .desktop file to tell plasma how they should be started, so we will start with that.
plasma-applet-tutorial1.desktop
[Desktop Entry]
Encoding=UTF-8
Name=Tutorial 1
Comment=Plasma Tutorial 1
Type=Service
ServiceTypes=Plasma/Applet
X-KDE-Library=plasma_applet_tutorial1
X-KDE-PluginInfo-Author=Bas Grolleman
[email protected]
X-KDE-PluginInfo-Name=plasma_applet_tutorial1
X-KDE-PluginInfo-Version=0.1
X-KDE-PluginInfo-Website=http://plasma.kde.org/
X-KDE-PluginInfo-Category=
X-KDE-PluginInfo-Depends=
X-KDE-PluginInfo-License=GPL
X-KDE-PluginInfo-EnabledByDefault=true
plasma-tutorial1.h
// Here we avoid loading the header multiple times
- ifndef Tutorial1_HEADER
- define Tutorial1_HEADER
// Ofcourse, we need the plasma headers
- include <Plasma/Applet>
class PlasmaTutorial1 : public Plasma::Applet
{
Q_OBJECT
public:
PlasmaTutorial1(QObject *parent, const QStringList &args);
~PlasmaTutorial1();
// This is the size of your applet
QRectF boundingRect() const;
// This paints your applet to the screen
void paintInterface(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget =0);
};
K_EXPORT_PLASMA_APPLET(tutorial1, PlasmaTutorial1)
- endif
plasma-tutorial1.cpp
- include "plasma-tutorial1.h"
- include <QPainter>
- include <QFontMetrics>
- include <KIcon>
PlasmaTutorial1::PlasmaTutorial1(QObject *parent, const QStringList &args)
: Plasma::Applet(parent, args)
{
}
PlasmaTutorial1::~PlasmaTutorial1()
{
}
QRectF PlasmaTutorial1::boundingRect() const
{
return QRectF(0,0,256,256);
}
void PlasmaTutorial1::paintInterface(QPainter *p, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
KIcon icon("document");
p->save();
p->setPen(Qt::black);
p->drawPixmap(0, 0, icon.pixmap(boundingRect().width()));
p->drawText(boundingRect(),Qt::AlignCenter,"Hello Plasmoid!");
p->restore();
}
- include "plasma-tutorial1.moc"