Development/Tutorials/Plasma4/GettingStarted: Difference between revisions
Bgrolleman (talk | contribs) m (→Abstract) |
Bgrolleman (talk | contribs) (Updating Plasma Tutorial, still need to add details to the .cpp file) |
||
Line 20: | Line 20: | ||
[[image:creatingyourfirstplasmoid1.png|frame|center]] | [[image:creatingyourfirstplasmoid1.png|frame|center]] | ||
==The Code== | == The Code == | ||
=== The .desktop file === | |||
Every Plasmoid needs a .desktop file to tell plasma how they should be started, and what name they carry. | |||
'''plasma-applet-tutorial1.desktop''' | '''plasma-applet-tutorial1.desktop''' | ||
Line 43: | Line 44: | ||
X-KDE-PluginInfo-EnabledByDefault=true | X-KDE-PluginInfo-EnabledByDefault=true | ||
</code> | </code> | ||
The most important bits are the '''X-KDE-Library''' and '''X-KDE-PluginInfo-Name''', they are the paste between your class and plasma, without it, nothing will start. | |||
=== The header file === | |||
This is the example header file, I will add lot's of comment's in the code to explain everything. | |||
'''plasma-tutorial1.h''' | '''plasma-tutorial1.h''' | ||
<code cppqt> | <code cppqt> | ||
// Here we avoid loading the header multiple times | // Here we avoid loading the header multiple times, this is required | ||
#ifndef Tutorial1_HEADER | #ifndef Tutorial1_HEADER | ||
#define Tutorial1_HEADER | #define Tutorial1_HEADER | ||
Line 52: | Line 58: | ||
#include <Plasma/Applet> | #include <Plasma/Applet> | ||
// Your Plasma Applet | |||
class PlasmaTutorial1 : public Plasma::Applet | class PlasmaTutorial1 : public Plasma::Applet | ||
{ | { | ||
Q_OBJECT | |||
public: | |||
// This is basic C++ , the create and destroy functions | |||
PlasmaTutorial1(QObject *parent, const QStringList &args); | |||
~PlasmaTutorial1(); | |||
// This is how plasma knows the size of your applet | |||
// if you have drawing issues (stuff staying behind for example) | |||
// than most times your drawing outside or at the border of this | |||
// rectangle | |||
QRectF boundingRect() const; | |||
// This is the main drawing function and used to paint your | |||
// plasmoid on screen | |||
void paintInterface(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget =0); | |||
}; | }; | ||
// This is the part that links your .desktop file. | |||
K_EXPORT_PLASMA_APPLET(tutorial1, PlasmaTutorial1) | K_EXPORT_PLASMA_APPLET(tutorial1, PlasmaTutorial1) | ||
#endif | #endif | ||
</code> | </code> | ||
{{tip| | |||
When using the K_EXPORT_PLASMA_APPLET do not start it with plasma_applet_ this is already done by the macro and doing it yourself will make a name diffrence between your .desktop and your libary, resulting in no plasmoid being started | |||
}} | |||
=== The actual work file === | |||
Here is the body of the function, again with a lot of comments in between. | |||
'''plasma-tutorial1.cpp''' | '''plasma-tutorial1.cpp''' | ||
<code cppqt> | <code cppqt> | ||
// We include the header and the required libaries | |||
#include "plasma-tutorial1.h" | #include "plasma-tutorial1.h" | ||
#include <QPainter> | #include <QPainter> |
Revision as of 06:16, 6 July 2007
Tutorial Series | Plasma Tutorial |
Previous | C++, Qt, KDE4 development environment |
What's Next | |
Further Reading | CMake |
Abstract
Where going to start creating a simple plasmoid in this tutorial, to keep things simple we will only create a static plasmoid, it will contain the following items.
- SVG Background
- Icon
- Some nice text
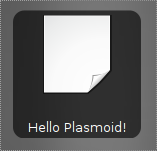
The Code
The .desktop file
Every Plasmoid needs a .desktop file to tell plasma how they should be started, and what name they carry.
plasma-applet-tutorial1.desktop
[Desktop Entry]
Encoding=UTF-8
Name=Tutorial 1
Comment=Plasma Tutorial 1
Type=Service
ServiceTypes=Plasma/Applet
X-KDE-Library=plasma_applet_tutorial1
X-KDE-PluginInfo-Author=Bas Grolleman
[email protected]
X-KDE-PluginInfo-Name=plasma_applet_tutorial1
X-KDE-PluginInfo-Version=0.1
X-KDE-PluginInfo-Website=http://plasma.kde.org/
X-KDE-PluginInfo-Category=
X-KDE-PluginInfo-Depends=
X-KDE-PluginInfo-License=GPL
X-KDE-PluginInfo-EnabledByDefault=true
The most important bits are the X-KDE-Library and X-KDE-PluginInfo-Name, they are the paste between your class and plasma, without it, nothing will start.
The header file
This is the example header file, I will add lot's of comment's in the code to explain everything.
plasma-tutorial1.h
// Here we avoid loading the header multiple times, this is required
- ifndef Tutorial1_HEADER
- define Tutorial1_HEADER
// Ofcourse, we need the plasma headers
- include <Plasma/Applet>
// Your Plasma Applet
class PlasmaTutorial1 : public Plasma::Applet
{
Q_OBJECT
public:
// This is basic C++ , the create and destroy functions
PlasmaTutorial1(QObject *parent, const QStringList &args);
~PlasmaTutorial1();
// This is how plasma knows the size of your applet
// if you have drawing issues (stuff staying behind for example)
// than most times your drawing outside or at the border of this
// rectangle
QRectF boundingRect() const;
// This is the main drawing function and used to paint your
// plasmoid on screen
void paintInterface(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget =0);
};
// This is the part that links your .desktop file.
K_EXPORT_PLASMA_APPLET(tutorial1, PlasmaTutorial1)
- endif
The actual work file
Here is the body of the function, again with a lot of comments in between.
plasma-tutorial1.cpp
// We include the header and the required libaries
- include "plasma-tutorial1.h"
- include <QPainter>
- include <QFontMetrics>
- include <KIcon>
PlasmaTutorial1::PlasmaTutorial1(QObject *parent, const QStringList &args)
: Plasma::Applet(parent, args)
{
}
PlasmaTutorial1::~PlasmaTutorial1()
{
}
QRectF PlasmaTutorial1::boundingRect() const
{
return QRectF(0,0,256,256);
}
void PlasmaTutorial1::paintInterface(QPainter *p, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
KIcon icon("document");
p->save();
p->setPen(Qt::black);
p->drawPixmap(0, 0, icon.pixmap(boundingRect().width()));
p->drawText(boundingRect(),Qt::AlignCenter,"Hello Plasmoid!");
p->restore();
}
- include "plasma-tutorial1.moc"