Development/Tutorials/Kate/KTextEditor Example
Parts to be reviewed:
- Port to KF5
- Update screenshot
Abstract
We build a small application using KTexteditor. This example supports syntax highlighting and other useful features. We see how to use KTextEditor.
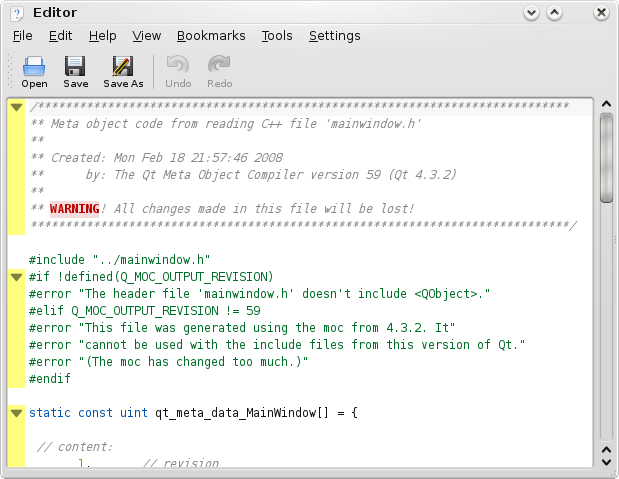
The Code
main.cpp
#include <KApplication>
#include <KAboutData>
#include <KCmdLineArgs>
#include "mainwindow.h"
int main (int argc, char *argv[])
{
KAboutData aboutData( "editor", "editor",
ki18n("Editor"), "1.0",
ki18n("A simple text area which can load and save."),
KAboutData::License_GPL,
ki18n("Copyright (c) 2007 Developer") );
KCmdLineArgs::init( argc, argv, &aboutData );
KApplication app;
MainWindow* window = new MainWindow();
window->show();
return app.exec();
}
In main.cpp just defines aboutData and app and shows Mainwindow.
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <KParts/MainWindow>
#include <QtGui/QKeyEvent>
namespace KTextEditor
{
class Document;
class View;
}
class MainWindow : public KParts::MainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent=0);
private slots:
void clear();
void openFile();
private:
void setupActions();
KTextEditor::View *m_view;
KTextEditor::Document *m_doc;
};
#endif
Class MainWindow is a successor of KXmlGuiWindow and contains KTextEditor (document and view) as a private Variable. There are also some useful methods defined.
mainwindow.cpp
#include "mainwindow.h"
#include <KApplication>
#include <KAction>
#include <KLocale>
#include <KActionCollection>
#include <KStandardAction>
#include <KFileDialog>
#include <KMessageBox>
#include <KIO/NetAccess>
#include <KSaveFile>
#include <QTextStream>
#include <KXMLGUIFactory>
#include <KTextEditor/Document>
#include <KTextEditor/View>
#include <KTextEditor/Editor>
#include <KTextEditor/EditorChooser>
MainWindow::MainWindow(QWidget *)
{
KTextEditor::Editor *editor = KTextEditor::EditorChooser::editor();
if (!editor) {
KMessageBox::error(this, i18n("A KDE text-editor component could not be found;\n"
"please check your KDE installation."));
kapp->exit(1);
}
m_doc = editor->createDocument(0);
m_view = qobject_cast<KTextEditor::View*>(m_doc->createView(this));
setCentralWidget(m_view);
setupActions();
setXMLFile("editorui.rc");
createShellGUI(true);
guiFactory()->addClient(m_view);
show ();
}
void MainWindow::setupActions()
{
KStandardAction::quit(kapp, SLOT(quit()), actionCollection());
KStandardAction::open(this, SLOT(openFile()), actionCollection());
KStandardAction::clear(this, SLOT(clear()), actionCollection());
}
void MainWindow::clear()
{
m_doc->clear();
}
void MainWindow::openFile()
{
m_view->document()->openUrl(KFileDialog::getOpenFileName());
}
The implementation is straight forward and self-explanatory. Some remarks:
MainWindow::Mainwindow()
First the editor component is created on the heap. After creating document() and view(), the GUI definitions are loaded from editorui.rc. The call guiFactory()->addClient(m_view) adds the kate parts menue and toolbar definitions to MainWindow. After that a full featured texteditor with syntax highlighting etc. is available for your application.
MainWindow::setupAction() defines three additional actions.
editorui.rc
<!DOCTYPE kpartgui SYSTEM "kpartgui.dtd">
<kpartgui name="editor" version="1"> <!-- increase version number if you don't see changes after experimenting with this file -->
<MenuBar>
<Menu name="file" noMerge="1"><text>&File</text>
<Action name="file_open" />
<DefineGroup name="save_merge" append="save_merge" />
<Separator/>
<DefineGroup name="revert_merge" append="revert_merge"/>
<DefineGroup name="print_merge" append="print_merge"/>
<Separator/>
<Action name="file_quit"/>
</Menu>
<Merge />
</MenuBar>
<ToolBar name="mainToolBar" noMerge="1"><text>Main Toolbar</text>
<Action name="file_open" />
<DefineGroup name="file_operations" />
<Separator />
<DefineGroup name="print_merge" />
<Separator />
<Action name="file_close" />
<Separator />
<DefineGroup name="edit_operations" />
<Separator />
<DefineGroup name="find_operations" />
<Separator />
<DefineGroup name="zoom_operations" />
</ToolBar>
<Menu name="ktexteditor_popup" noMerge="1">
<DefineGroup name="popup_operations" />
</Menu>
</kpartgui>
CMakeLists.txt
project(editor)
find_package(KDE4 REQUIRED)
include_directories(${KDE4_INCLUDES})
set(editor_SRCS
main.cpp
mainwindow.cpp
)
kde4_add_executable(editor ${editor_SRCS})
target_link_libraries(editor ${KDE4_KDEUI_LIBS}
${KDE4_KIO_LIBS}
${KDE4_KTEXTEDITOR_LIBS})
install(TARGETS editor DESTINATION ${BIN_INSTALL_DIR})
install(FILES editorui.rc
DESTINATION ${DATA_INSTALL_DIR}/editor)